Hướng dẫn và ví dụ Android TextWatcher
1. Android TextWatcher
Như bạn đã biết TextEdit cho phép người dùng nhập và sửa đổi văn bản. TextEdit sử dụng interface TextWatcher để xem các thay đổi xẩy ra đối với văn bản của nó, hoặc sửa đổi nội dung văn bản.
editText.addTextChangedListener(TextWatcher watcher)editText.addTextChangedListener(TextWatcher watcher)
Các phương thức của interface TextWatcher:
- void afterTextChanged(Editable s)
- void beforeTextChanged(CharSequence s, int start, int count, int after)
- void onTextChanged(CharSequence s, int start, int before, int count)
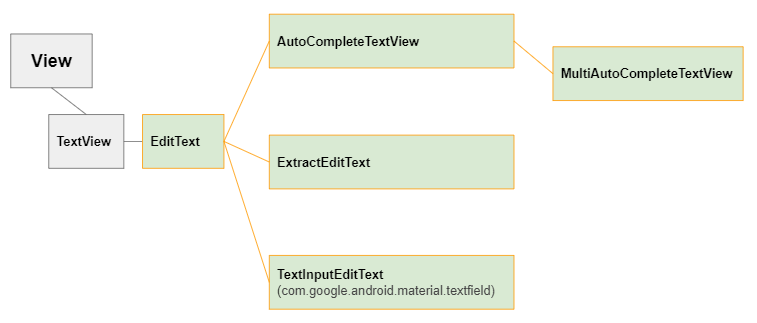
TextWatcher có thể được sử dụng để đảm bảo rằng người dùng sẽ nhập vào một văn bản phù hợp với một khuôn mẫu (pattern) cho trước.
3. Example: Date Pattern
Trong ví dụ này chúng ta sẽ sử dụng TextWatcher để đảm bảo rằng người dùng sẽ nhập vào một ngày tháng (date) hợp lệ theo định dạng DD/MM/YYYY.
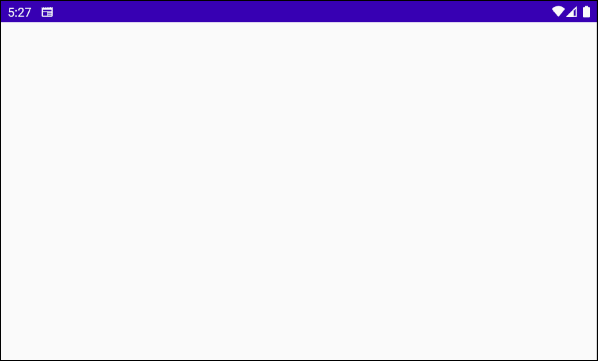
DateFormatTextWatcher.java
package org.o7planning.textwatcherdateexample;
import android.text.Editable;
import android.text.TextWatcher;
import android.widget.EditText;
import java.util.Calendar;
public class DateFormatTextWatcher implements TextWatcher {
private static final String DDMMYYYY = "DDMMYYYY";
private static final String SEPARATOR = "/";
private final Calendar calendar = Calendar.getInstance();
private String currentText = "";
private EditText editText;
public DateFormatTextWatcher(EditText editText) {
this.editText = editText;
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
if (!s.toString().equals(this.currentText)) {
// Remove all non-digit.
String newTextClean = s.toString().replaceAll("[^\\d.]|\\.", "");
String currentTextClean = this.currentText.replaceAll("[^\\d.]|\\.", "");
int newTextLength = newTextClean.length();
// Cursor Position Index.
int selectionIndex = newTextLength;
for (int i = 2; i <= newTextLength && i < 6; i += 2) {
selectionIndex++;
}
// Fix for pressing delete next to a forward slash
if (newTextClean.equals(currentTextClean)) {
selectionIndex--;
}
if (newTextClean.length() < 8) {
newTextClean = newTextClean + this.DDMMYYYY.substring(newTextClean.length());
} else {
// This part makes sure that when we finish entering numbers
// the date is correct, fixing it otherwise
int day = Integer.parseInt(newTextClean.substring(0,2));
int month = Integer.parseInt(newTextClean.substring(2,4));
int year = Integer.parseInt(newTextClean.substring(4,8));
month = month < 1 ? 1 : month > 12 ? 12 : month;
this.calendar.set(Calendar.MONTH, month-1);
year = (year < 1900)? 1900:(year > 2100)? 2100 : year;
this.calendar.set(Calendar.YEAR, year);
// ^ first set year for the line below to work correctly
// with leap years - otherwise, date e.g. 29/02/2012
// would be automatically corrected to 28/02/2012
day = (day > this.calendar.getActualMaximum(Calendar.DATE))? this.calendar.getActualMaximum(Calendar.DATE):day;
newTextClean = String.format("%02d%02d%02d",day, month, year);
}
// "%s/%s/%s"
String format = "%s" + SEPARATOR + "%s" + SEPARATOR +"%s";
newTextClean = String.format(format, newTextClean.substring(0, 2),
newTextClean.substring(2, 4),
newTextClean.substring(4, 8));
selectionIndex = selectionIndex < 0 ? 0 : selectionIndex;
this.currentText = newTextClean;
this.editText.setText(this.currentText);
this.editText.setSelection(selectionIndex < this.currentText.length() ? selectionIndex : this.currentText.length());
}
}
@Override
public void afterTextChanged(Editable s) {
}
}
Giao diện của ví dụ:
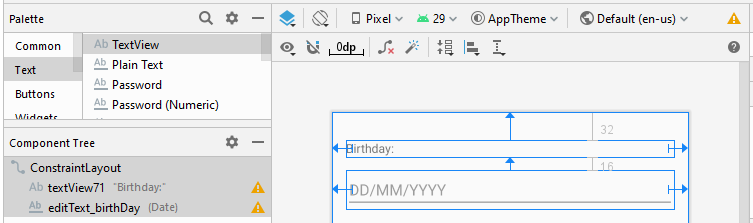
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView71"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Birthday:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_birthDay"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:hint="DD/MM/YYYY"
android:inputType="date"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView71" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.textwatcherdateexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.TextWatcher;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
private EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editText = (EditText) this.findViewById(R.id.editText_birthDay);
// Create TextWatcher:
TextWatcher textWatcher = new DateFormatTextWatcher(this.editText);
this.editText.addTextChangedListener(textWatcher);
}
}
4. Example: Number
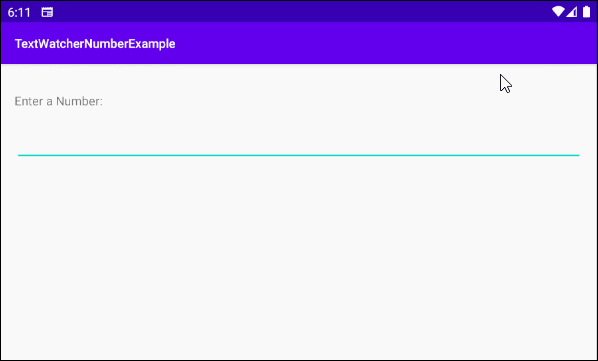
NumberTextWatcher.java
package org.o7planning.textwatchernumberexample;
import android.text.Editable;
import android.text.TextWatcher;
import android.text.method.DigitsKeyListener;
import android.util.Log;
import android.widget.EditText;
import java.math.RoundingMode;
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.text.ParseException;
import java.util.Locale;
public class NumberTextWatcher implements TextWatcher {
private static final String LOG_TAG = "AndroidExample";
private final int numDecimals;
private String groupingSeparator;
private String decimalSeparator;
private boolean nonUsFormat;
private DecimalFormat decimalFormatDec;
private DecimalFormat decimalFormatInt;
private boolean hasFractionalPart;
private EditText editText;
private String value;
public NumberTextWatcher(EditText editText, Locale locale, int numDecimals) {
this.editText = editText;
this.numDecimals = numDecimals;
this.hasFractionalPart = false;
this.editText.setKeyListener(DigitsKeyListener.getInstance("0123456789.,"));
DecimalFormatSymbols symbols = new DecimalFormatSymbols(locale);
char gs = symbols.getGroupingSeparator();
char ds = symbols.getDecimalSeparator();
this.groupingSeparator = String.valueOf(gs);
this.decimalSeparator = String.valueOf(ds);
String patternInt = "#,###";
this.decimalFormatInt = new DecimalFormat(patternInt, symbols);
String patternDec = patternInt + "." + replicate('#', this.numDecimals);
this.decimalFormatDec = new DecimalFormat(patternDec, symbols);
this.decimalFormatDec.setDecimalSeparatorAlwaysShown(true);
this.decimalFormatDec.setRoundingMode(RoundingMode.DOWN);
this.nonUsFormat = !this.decimalSeparator.equals(".");
this.value = null;
}
@Override
public void afterTextChanged(Editable s) {
Log.d(LOG_TAG, "afterTextChanged");
this.editText.removeTextChangedListener(this);
try {
int initLeng = this.editText.getText().length();
String v = this.value.replace(this.groupingSeparator, "");
Number n = this.decimalFormatDec.parse(v);
int selectionStart = this.editText.getSelectionStart();
if (this.hasFractionalPart) {
int decPos = v.indexOf(this.decimalSeparator) + 1;
int decLen = v.length() - decPos;
if (decLen > this.numDecimals) {
v = v.substring(0, decPos + this.numDecimals);
}
int trz = countTrailingZeros(v);
StringBuilder fmt = new StringBuilder(this.decimalFormatDec.format(n));
while (trz-- > 0) {
fmt.append("0");
}
this.editText.setText(fmt.toString());
} else {
this.editText.setText(this.decimalFormatInt.format(n));
}
int endLeng = this.editText.getText().length();
int selection = (selectionStart + (endLeng - initLeng));
if (selection > 0 && selection <= this.editText.getText().length()) {
this.editText.setSelection(selection);
} else {
// Place cursor at the end?
this.editText.setSelection(this.editText.getText().length() - 1);
}
} catch (NumberFormatException | ParseException nfe) {
// Do nothing?
}
this.editText.addTextChangedListener(this);
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
Log.d(LOG_TAG, "beforeTextChanged");
this.value = this.editText.getText().toString();
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
Log.d(LOG_TAG, "onTextChanged");
String newValue = s.toString();
String change = newValue.substring(start, start + count);
String prefix = this.value.substring(0, start);
String suffix = this.value.substring(start + before);
if (".".equals(change) && this.nonUsFormat) {
change = this.decimalSeparator;
}
this.value = prefix + change + suffix;
this.hasFractionalPart = this.value.contains(this.decimalSeparator);
Log.d(LOG_TAG, "VALUE: " + this.value);
}
private int countTrailingZeros(String str) {
int count = 0;
for (int i = str.length() - 1; i >= 0; i--) {
char ch = str.charAt(i);
if ('0' == ch) {
count++;
} else {
break;
}
}
return count;
}
private String replicate(char ch, int n) {
return new String(new char[n]).replace("\0", "" + ch);
}
}
Giao diện của ví dụ:
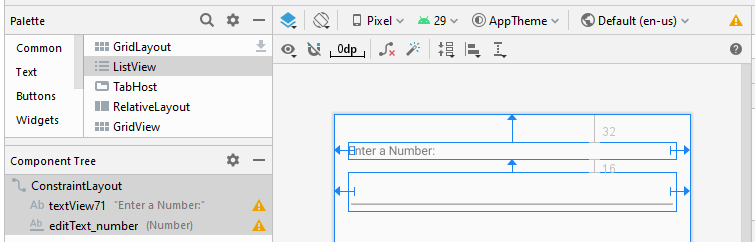
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView71"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginLeft="16dp"
android:layout_marginTop="32dp"
android:layout_marginRight="16dp"
android:text="Enter a Number:"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_number"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView71" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.textwatchernumberexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.TextWatcher;
import android.widget.EditText;
import java.util.Locale;
public class MainActivity extends AppCompatActivity {
private EditText editTextNumber;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextNumber = (EditText) this.findViewById(R.id.editText_number) ;
Locale locale = new Locale("en", "US");
int numDecs = 2; // Let's use 2 decimals
TextWatcher textWatcher = new NumberTextWatcher(this.editTextNumber, locale, numDecs);
this.editTextNumber.addTextChangedListener(textWatcher);
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More