Hướng dẫn và ví dụ Android SMS
1. Gửi SMS trong Android
Trong Android, về cơ bản có 2 cách để bạn gửi một tin nhắn SMS đó là sử dụng SmsManager và Intent.ACTION_SEND.
SmsManager
SmsManager cho phép bạn gửi tin nhắn SMS một cách trực tiếp từ ứng dụng của bạn tới một số điện thoại bất kỳ.
Intent.ACTION_SEND
Từ ứng dụng của bạn, bạn có thể tạo một Intent không tường minh (implicit Intent) gửi đến hệ thống Android để yêu cầu hệ thống gửi tin nhắn giúp bạn. Hệ thống sẽ mở ra một ứng dụng gửi SMS tốt nhất sẵn có trên hệ thống để làm việc này, và nhiều khả năng nó sẽ sử dụng ứng dụng Messenger.
Ứng dụng Messenger chính là ứng dụng gửi SMS có sẵn trên Android mà tất cả người dùng đều quen thuộc với nó.
Nếu bạn test các ứng dụng gửi SMS trên Android Emulator (Trình giả lập Android) bạn cần biết số điện thoại của nó, và bạn có thể test ứng dụng bằng cách gửi SMS vào số điện thoại này.
2. Gửi SMS với SmsManager
SmsManager là một lớp có sẵn trong Android, nó cho phép bạn gửi trực tiếp một SMS tới một số điện thoại bất kỳ.
Trong ví dụ này tôi sẽ gửi tin nhắn SMS tới số điện thoại của chính thiết bị đang test, vì vậy ngay sau khi nhấn vào nút gửi tin nhắn sẽ thấy thông báo nhận được một SMS. Xem trước ví dụ:
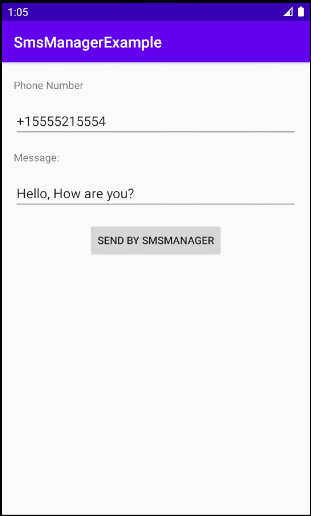
Chú ý: Nếu người dùng đã cho phép ứng dụng gửi tin nhắn SMS, ứng dụng sẽ không cần hỏi lại người dùng trong các lần gửi SMS tiếp theo, vì vậy đôi khi bạn phải loại bỏ các quyền (permission) đã cấp cho ứng dụng để có thể test ứng dụng một cách đầy đủ hơn.
OK, Trên Androidi Studio tạo một project:
- Name: SmsManagerExample
- Package name: org.o7planning.smsmanagerexample
Thêm một đoạn mã XML dưới đây vào tập tin AndroidManifest.xml để cho phép ứng dụng gửi SMS. Chú ý: Với Android 6.0+ (API Level 23+) ứng dụng của bạn cần phải hỏi người dùng để được phép gửi SMS (Xem thêm trong code của ví dụ).
<uses-permission android:name="android.permission.SEND_SMS"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.smsmanagerexample">
<uses-permission android:name="android.permission.SEND_SMS"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Giao diện của ứng dụng:
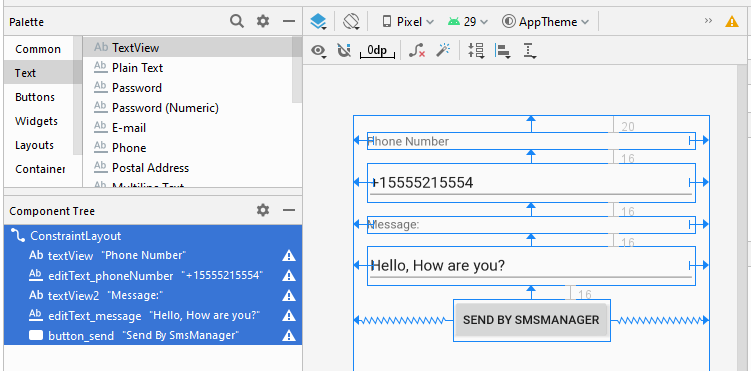
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Phone Number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_phoneNumber"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:hint="Phone Number"
android:inputType="phone"
android:text="+15555215554"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Message:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_phoneNumber" />
<EditText
android:id="@+id/editText_message"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="text"
android:text="Hello, How are you?"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<Button
android:id="@+id/button_send"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Send By SmsManager"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_message" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.xml
package org.o7planning.smsmanagerexample;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.telephony.SmsManager;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final int MY_PERMISSION_REQUEST_CODE_SEND_SMS = 1;
private static final String LOG_TAG = "AndroidExample";
private EditText editTextPhoneNumber;
private EditText editTextMessage;
private Button buttonSend;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextPhoneNumber = (EditText) this.findViewById(R.id.editText_phoneNumber);
this.editTextMessage = (EditText) this.findViewById(R.id.editText_message);
this.buttonSend = (Button) this.findViewById(R.id.button_send);
this.buttonSend.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
askPermissionAndSendSMS();
}
});
}
private void askPermissionAndSendSMS() {
// With Android Level >= 23, you have to ask the user
// for permission to send SMS.
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.M) { // 23
// Check if we have send SMS permission
int sendSmsPermisson = ActivityCompat.checkSelfPermission(this,
Manifest.permission.SEND_SMS);
if (sendSmsPermisson != PackageManager.PERMISSION_GRANTED) {
// If don't have permission so prompt the user.
this.requestPermissions(
new String[]{Manifest.permission.SEND_SMS},
MY_PERMISSION_REQUEST_CODE_SEND_SMS
);
return;
}
}
this.sendSMS_by_smsManager();
}
private void sendSMS_by_smsManager() {
String phoneNumber = this.editTextPhoneNumber.getText().toString();
String message = this.editTextMessage.getText().toString();
try {
// Get the default instance of the SmsManager
SmsManager smsManager = SmsManager.getDefault();
// Send Message
smsManager.sendTextMessage(phoneNumber,
null,
message,
null,
null);
Log.i( LOG_TAG,"Your sms has successfully sent!");
Toast.makeText(getApplicationContext(),"Your sms has successfully sent!",
Toast.LENGTH_LONG).show();
} catch (Exception ex) {
Log.e( LOG_TAG,"Your sms has failed...", ex);
Toast.makeText(getApplicationContext(),"Your sms has failed... " + ex.getMessage(),
Toast.LENGTH_LONG).show();
ex.printStackTrace();
}
}
// When you have the request results
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case MY_PERMISSION_REQUEST_CODE_SEND_SMS: {
// Note: If request is cancelled, the result arrays are empty.
// Permissions granted (SEND_SMS).
if (grantResults.length > 0
&& grantResults[0] == PackageManager.PERMISSION_GRANTED) {
Log.i( LOG_TAG,"Permission granted!");
Toast.makeText(this, "Permission granted!", Toast.LENGTH_LONG).show();
this.sendSMS_by_smsManager();
}
// Cancelled or denied.
else {
Log.i( LOG_TAG,"Permission denied!");
Toast.makeText(this, "Permission denied!", Toast.LENGTH_LONG).show();
}
break;
}
}
}
// When results returned
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == MY_PERMISSION_REQUEST_CODE_SEND_SMS) {
if (resultCode == RESULT_OK) {
// Do something with data (Result returned).
Toast.makeText(this, "Action OK", Toast.LENGTH_LONG).show();
} else if (resultCode == RESULT_CANCELED) {
Toast.makeText(this, "Action canceled", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Action Failed", Toast.LENGTH_LONG).show();
}
}
}
}
3. Gửi SMS với Intent.ACTION_SEND
Từ ứng dụng của bạn, bạn có thể tạo một Intent không tường minh (implicit Intent) gửi đến hệ thống Android để yêu cầu hệ thống gửi tin nhắn giúp bạn. Hệ thống sẽ mở ra một ứng dụng gửi SMS tốt nhất sẵn có trên hệ thống để làm việc này, và nhiều khả năng nó sẽ sử dụng ứng dụng Messenger.
Ứng dụng Messenger chính là ứng dụng gửi SMS có sẵn trên Android mà tất cả người dùng đều quen thuộc với nó.
Xem trước ví dụ:
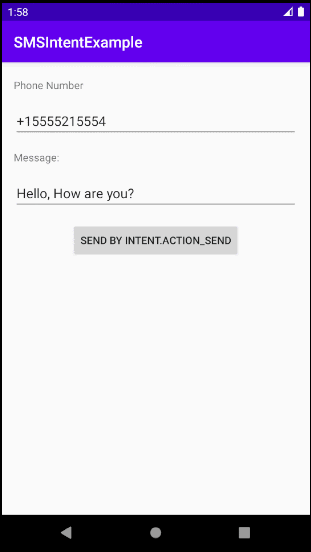
Trên Android Studio tạo một project:
- Name: SMSIntentExample
- Package name: org.o7planning.smsintentexample
Thêm một đoạn mã XML dưới đây vào tập tin AndroidManifest.xml để cho phép ứng dụng gửi SMS.
<uses-permission android:name="android.permission.SEND_SMS"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.smsintentexample">
<uses-permission android:name="android.permission.SEND_SMS"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Giao diện của ứng dụng ví dụ:
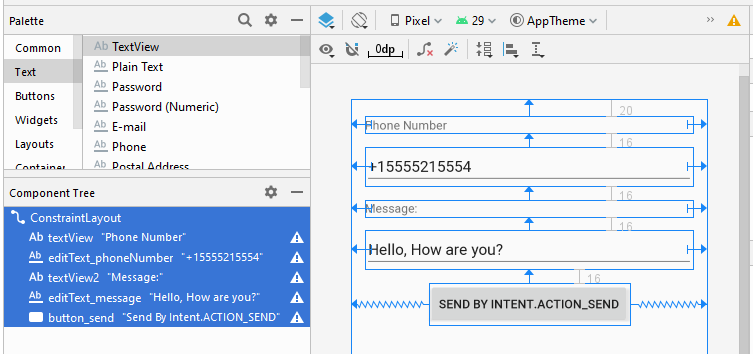
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Phone Number"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_phoneNumber"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:hint="Phone Number"
android:inputType="phone"
android:text="+15555215554"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Message:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_phoneNumber" />
<EditText
android:id="@+id/editText_message"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="text"
android:text="Hello, How are you?"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2" />
<Button
android:id="@+id/button_send"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Send By Intent.ACTION_SEND"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_message" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.smsintentexample;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private static final String LOG_TAG = "AndroidExample";
private EditText editTextPhoneNumber;
private EditText editTextMessage;
private Button buttonSend;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextPhoneNumber = (EditText) this.findViewById(R.id.editText_phoneNumber);
this.editTextMessage = (EditText) this.findViewById(R.id.editText_message);
this.buttonSend = (Button) this.findViewById(R.id.button_send);
this.buttonSend.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sendSMS_by_Intent_ACTION_SEND();
}
});
}
private void sendSMS_by_Intent_ACTION_SEND() {
String phoneNumber = this.editTextPhoneNumber.getText().toString();
String message = this.editTextMessage.getText().toString();
// Add the phone number in the data
Uri uri = Uri.parse("smsto:" + phoneNumber);
Intent smsIntent = new Intent(Intent.ACTION_SENDTO, uri);
// Add the message at the sms_body extra field
smsIntent.putExtra("sms_body", message);
try {
startActivity(smsIntent);
} catch (Exception ex) {
Log.e(LOG_TAG, "Your sms has failed... " + ex.getMessage(), ex);
Toast.makeText(MainActivity.this, "Your sms has failed... " + ex.getMessage(),
Toast.LENGTH_LONG).show();
ex.printStackTrace();
}
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More