Tạo một File Finder Dialog đơn giản trong Android
1. Mục tiêu của ví dụ
Trong bài viết này tôi sẽ hướng dẫn bạn tạo một ứng dụng đơn giản, tìm kiếm các tập tin trong Android và hiển thị kết quả trên một Dialog.
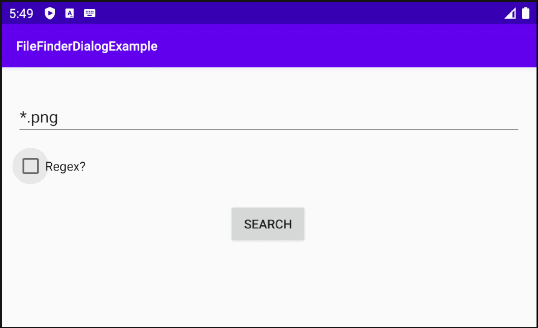
Ứng dụng này sẽ tìm kiếm các tập tin trên SD Card (Thẻ SD), vì vậy nếu bạn test ứng dụng này trên Android Emulator bạn cần phải thiết lập SD Card cho nó.
Sử dụng Device File Explorer để copy một vài tập tin vào Android Emulator, điều này là cần thiết để bạn test ứng dụng.
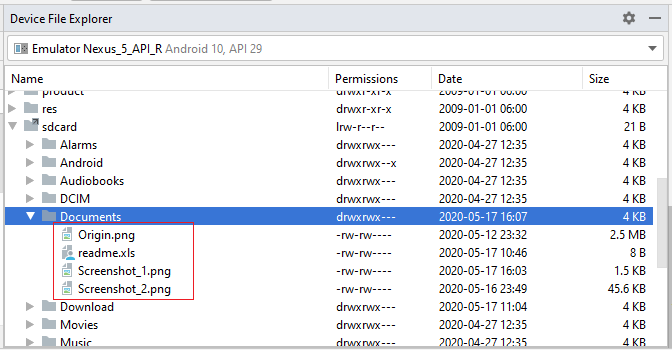
2. Ví dụ File Finder Dialog
Trên Android Studio tạo mới một project:
- File > New > New Project > Empty Activity
- Name: FileFinderDialogExample
- Package name: org.o7planning.filefinderdialogexample
- Language: Java
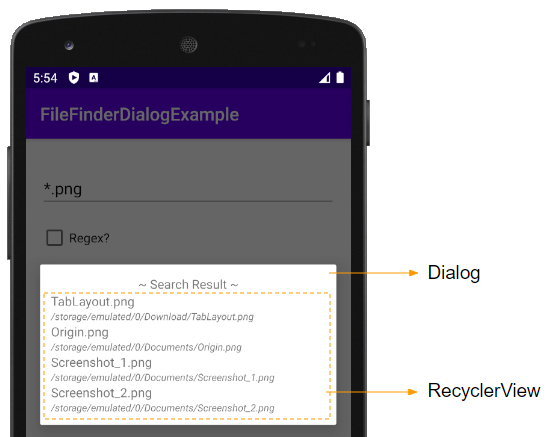
Sau khi người dùng nhấn vào nút tìm kiếm, danh sách các tập tin tìm thấy sẽ được hiển thị trên một RecyclerView. Đây là một thành phần giao diện không sẵn có trên Android SDK vì vậy bạn phải cài đặt nó vào project.
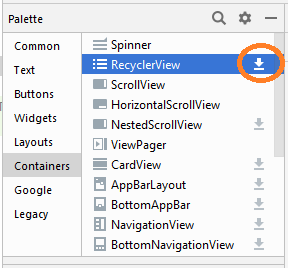
Sau khi cài đặt thư viện thành công bạn sẽ nhìn thấy nó được khai báo trong build.gradle (Module App):
dependencies {
...
implementation 'androidx.recyclerview:recyclerview:1.0.0'
}
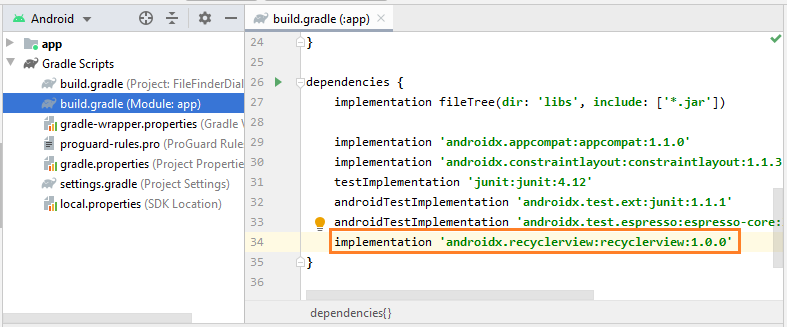
Tiếp theo, đăng ký với hệ thống để ứng dụng của bạn được phép truy cập vào các tập tin.
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="org.o7planning.filefinderdialogexample">
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Giao diện của ứng dụng:
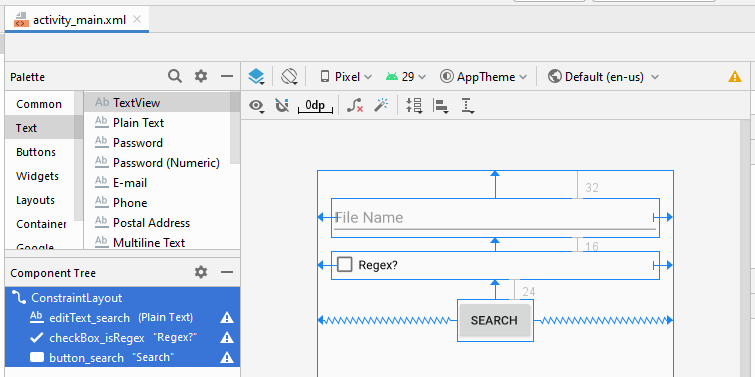
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText_search"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:hint="File Name"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<CheckBox
android:id="@+id/checkBox_isRegex"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Regex?"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_search" />
<Button
android:id="@+id/button_search"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:text="Search"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_isRegex" />
</androidx.constraintlayout.widget.ConstraintLayout>
Lớp FileFinder bao gồm các phương thức để tìm kiếm các tập tin trong hệ thống. Chú ý: Với Android 6.0+ (API Level 23+) ứng dụng của bạn cần phải hỏi người dùng để được phép truy cập vào các tập tin, nếu không được phép của người dùng các phương thức này sẽ luôn trả về một danh sách rỗng.
FileFinder.java
package org.o7planning.filefinderdialogexample;
import android.os.Environment;
import android.util.Log;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Pattern;
public class FileFinder {
private static final String LOG_TAG_FILE_FINDER = "FileSelector";
private boolean enableLog;
public FileFinder(boolean enableLog) {
this.enableLog = enableLog;
}
public List<File> findByKeyword(File rootDir, String keywordFileName) {
if(keywordFileName==null || keywordFileName.isEmpty()) {
return new ArrayList<File>();
}
String regexFileName = keywordFileName.replace("*", ".*?");
List<File> resultList = this.listOfFile(rootDir, regexFileName);
return resultList;
}
public List<File> findByRegex(File rootDir, String regexFileName) {
List<File> resultList = this.listOfFile(rootDir, regexFileName);
return resultList;
}
public List<File> findInSDCardByKeyword(String keywordFileName) {
// (Example): /storage/emulated/0
String sdCardPath = Environment.getExternalStorageDirectory().getAbsolutePath();
this.log("External Storage Directory: " + sdCardPath);
File sdCardDir = new File(sdCardPath);
return this.findByKeyword(sdCardDir, keywordFileName);
}
public List<File> findInSDCardByRegex(String regexFileName) {
// (Example): /storage/emulated/0
String sdCardPath = Environment.getExternalStorageDirectory().getAbsolutePath();
this.log("External Storage Directory: " + sdCardPath);
File sdCardDir = new File(sdCardPath);
return this.findByRegex(sdCardDir, regexFileName);
}
private List<File> listOfFile(File dir, String regexFileName) {
Pattern patternFileName = Pattern.compile(regexFileName);
List<File> resultList = new ArrayList<File>();
this.listOfFile(dir, patternFileName, resultList);
return resultList;
}
private void listOfFile(File dir, Pattern patternFileName, List<File> resultList) {
this.log("LIST OF DIR " + dir.getAbsolutePath());
File[] list = dir.listFiles();
if(list == null) {
this.log("Directory" + dir.getAbsolutePath()+ " has no files");
return;
}
this.log("Directory" + dir.getAbsolutePath()+ " has " + list.length +" direct files");
for (File file : list) {
if (file.isDirectory()) {
if (!new File(file, ".nomedia").exists() && !file.getName().startsWith(".")) {
this.log( "IS DIR " + file);
listOfFile(file, patternFileName, resultList);
}
} else {
String path = file.getAbsolutePath();
this.log( "FILE PATH: " + path);
String fileName = file.getName();
if(patternFileName.matcher(fileName).find()) {
resultList.add(file);
this.log( "ADD " + path);
}
}
}
}
private void log(String message) {
if(enableLog) {
Log.i(LOG_TAG_FILE_FINDER, message);
}
}
}
OnFileSelectListener.java
package org.o7planning.filefinderdialogexample;
import java.io.File;
public interface OnFileSelectListener {
void onSelect(File file);
}
ResultDialog.java
package org.o7planning.filefinderdialogexample;
import android.app.Dialog;
import android.content.Context;
import android.graphics.Typeface;
import android.util.TypedValue;
import android.view.Gravity;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.LinearLayout;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import java.io.File;
import java.util.List;
public class ResultDialog extends Dialog {
public ResultDialog(@NonNull Context context, List<File> resultList, OnFileSelectListener listener) {
super(context);
this.requestWindowFeature(Window.FEATURE_NO_TITLE);
//
LinearLayout linearLayout = new LinearLayout(this.getContext());
linearLayout.setLayoutParams(new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT));
linearLayout.setOrientation(LinearLayout.VERTICAL);
int p = convertToPixels(12);
linearLayout.setPadding(p, p, p, p);
linearLayout.setGravity(Gravity.CENTER);
TextView textView = new TextView(this.getContext());
textView.setLayoutParams(new LinearLayout.LayoutParams(screenWidth(), ViewGroup.LayoutParams.WRAP_CONTENT));
textView.setGravity(Gravity.CENTER);
textView.setText("~ Search Result ~");
RecyclerView recyclerView = new RecyclerView(this.getContext());
linearLayout.addView(textView);
linearLayout.addView(recyclerView);
RecyclerViewAdapter adapter = new RecyclerViewAdapter(this.getContext(), this, listener, resultList);
recyclerView.setAdapter(adapter);
LinearLayoutManager layoutManager = new LinearLayoutManager(this.getContext());
layoutManager.setOrientation(LinearLayoutManager.VERTICAL);
recyclerView.setLayoutManager(layoutManager);
//
// this.getWindow().setBackgroundDrawable(new ColorDrawable(android.graphics.Color.TRANSPARENT));
//
this.setContentView(linearLayout);
this.setCancelable(true);
}
private int convertToPixels(int dp) {
float scale = this.getContext().getResources().getDisplayMetrics().density;
return (int) (dp * scale + 0.5f);
}
private int screenWidth() {
return this.getContext().getResources().getDisplayMetrics().widthPixels;
}
// RecyclerViewAdapter
private class RecyclerViewAdapter extends RecyclerView.Adapter<RecyclerViewAdapter.ViewHolder> {
private Context context;
private List<File> files;
private OnFileSelectListener listener;
private Dialog dialog;
public RecyclerViewAdapter(Context context, Dialog dialog, OnFileSelectListener listener, List<File> files) {
this.context = context;
this.files = files;
this.listener = listener;
this.dialog = dialog;
}
// Create new views (invoked by the layout manager)
@Override
public RecyclerViewAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
LinearLayout linearLayout = new LinearLayout(context);
linearLayout.setOrientation(LinearLayout.VERTICAL);
TextView txtName = new TextView(context);
TextView txtPath = new TextView(context);
txtPath.setTypeface(txtPath.getTypeface(), Typeface.ITALIC);
txtPath.setTextSize(TypedValue.COMPLEX_UNIT_SP, 11);
linearLayout.addView(txtName);
linearLayout.addView(txtPath);
RecyclerViewAdapter.ViewHolder viewHolder = new RecyclerViewAdapter.ViewHolder(linearLayout);
return viewHolder;
}
// Inner class to hold a reference to each item of RecyclerView
public class ViewHolder extends RecyclerView.ViewHolder {
public LinearLayout linearLayout;
public TextView textViewFileName;
public TextView textViewPath;
public ViewHolder(View itemLayoutView) {
super(itemLayoutView);
this.linearLayout = (LinearLayout) itemLayoutView;
this.textViewFileName = (TextView) linearLayout.getChildAt(0);
this.textViewPath = (TextView) linearLayout.getChildAt(1);
}
}
@Override
public int getItemCount() {
return files.size();
}
@Override
public void onBindViewHolder(RecyclerViewAdapter.ViewHolder viewHolder, final int position) {
final File selectedFile = files.get(position);
final String path = selectedFile.getAbsolutePath();
String[] split = path.split("/");
final String name = split[split.length - 1];
viewHolder.textViewFileName.setText(name);
viewHolder.textViewPath.setText(path);
viewHolder.linearLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dialog.dismiss();
listener.onSelect(selectedFile);
}
});
}
}
}
MainActivity.java
package org.o7planning.filefinderdialogexample;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.Toast;
import java.io.File;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private static final int MY_REQUEST_CODE_PERMISSION = 1000;
private static final String LOG_TAG = "AndroidExample";
private Button buttonSearch;
private EditText editTextSearch;
private CheckBox checkBoxIsRegex;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextSearch = (EditText) this.findViewById(R.id.editText_search);
this.checkBoxIsRegex = (CheckBox) this.findViewById(R.id.checkBox_isRegex);
this.buttonSearch = (Button) this.findViewById(R.id.button_search);
this.buttonSearch.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v) {
askPermissionAndSearchFile();
}
});
}
private void askPermissionAndSearchFile() {
// With Android Level >= 23, you have to ask the user
// for permission to access External Storage.
if (android.os.Build.VERSION.SDK_INT >= android.os.Build.VERSION_CODES.M) { // Level 23
// Check if we have Call permission
int permisson = ActivityCompat.checkSelfPermission(this,
Manifest.permission.READ_EXTERNAL_STORAGE);
if (permisson != PackageManager.PERMISSION_GRANTED) {
// If don't have permission so prompt the user.
this.requestPermissions(
new String[]{Manifest.permission.READ_EXTERNAL_STORAGE},
MY_REQUEST_CODE_PERMISSION
);
return;
}
}
this.doSearchFile();
}
private void doSearchFile() {
FileFinder fileSelector = new FileFinder(true);
String searchText = this.editTextSearch.getText().toString();
boolean isRegex = this.checkBoxIsRegex.isChecked();
List<File> resultList = null;
if(isRegex) {
resultList = fileSelector.findInSDCardByRegex(searchText);
} else {
resultList = fileSelector.findInSDCardByKeyword(searchText);
}
ResultDialog dialog = new ResultDialog(this, resultList, new OnFileSelectListener() {
@Override
public void onSelect(File file) {
Toast.makeText(MainActivity.this, "Path: " + file.getAbsolutePath(), Toast.LENGTH_LONG).show();
}
});
dialog.show();
}
// When you have the request results
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
//
switch (requestCode) {
case MY_REQUEST_CODE_PERMISSION: {
// Note: If request is cancelled, the result arrays are empty.
// Permissions granted (CALL_PHONE).
if (grantResults.length > 0
&& grantResults[0] == PackageManager.PERMISSION_GRANTED) {
Log.i( LOG_TAG,"Permission granted!");
Toast.makeText(this, "Permission granted!", Toast.LENGTH_SHORT).show();
this.doSearchFile();
}
// Cancelled or denied.
else {
Log.i(LOG_TAG,"Permission denied!");
Toast.makeText(this, "Permission denied!", Toast.LENGTH_SHORT).show();
}
break;
}
}
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More