Hướng dẫn xử lý JSON trong Android
1. JSON là gì?
JSON (JavaScript Object Notation) là một định dạng trao đổi dữ liệu dữ liệu (data exchange format). Nó lưu trữ các dữ liệu theo cặp khóa và giá trị. So với XML thì JSON đơn giản về dễ đọc hơn.
Hãy xem một ví dụ đơn giản của JSON:
{
"name" : "Tran",
"address" : "Hai Duong, Vietnam",
"phones" : [0121111111, 012222222]
}
Các cặp key-value có thể lồng nhau:
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
Trong Java có rất nhiều các thư viện mã nguồn mở giúp bạn thao tác với tài liệu JSON, chẳng hạn như:
- JSONP
- json.org
- Jackson
- Google GSON
- json-lib
- javax json
- json-simple
- json-smart
- flexjson
- fastjson
Android hỗ trợ sẵn thư viện để làm việc với JSON, bạn không cần phải khai báo bất cứ một thư viện nào khác. Trong tài liệu hướng dẫn này tôi sẽ hướng dẫn bạn làm việc với JSON sử dụng JSON API có sẵn trong hệ điều hành của Android.
3. Tạo nhanh một Project để làm việc với JSON
Tôi tạo nhanh một project có tên JSONTutorial để làm việc với JSON thông qua các ví dụ.
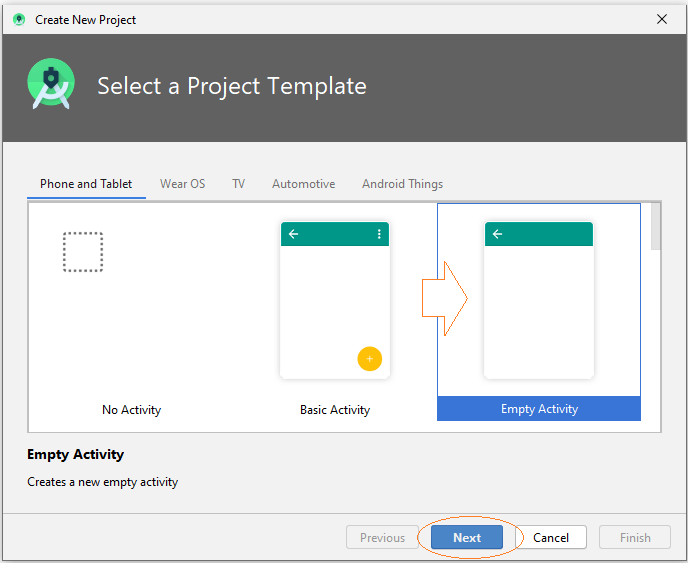
- Name: JSONTutorial
- Package name: org.o7planning.jsontutorial
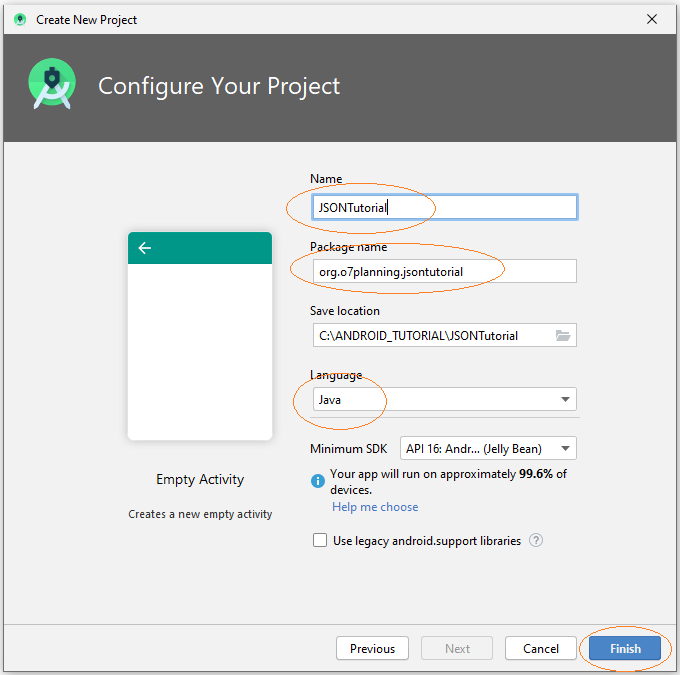
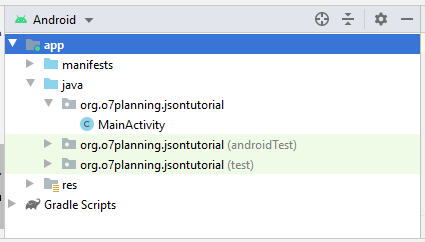
Tạo thư mục raw:
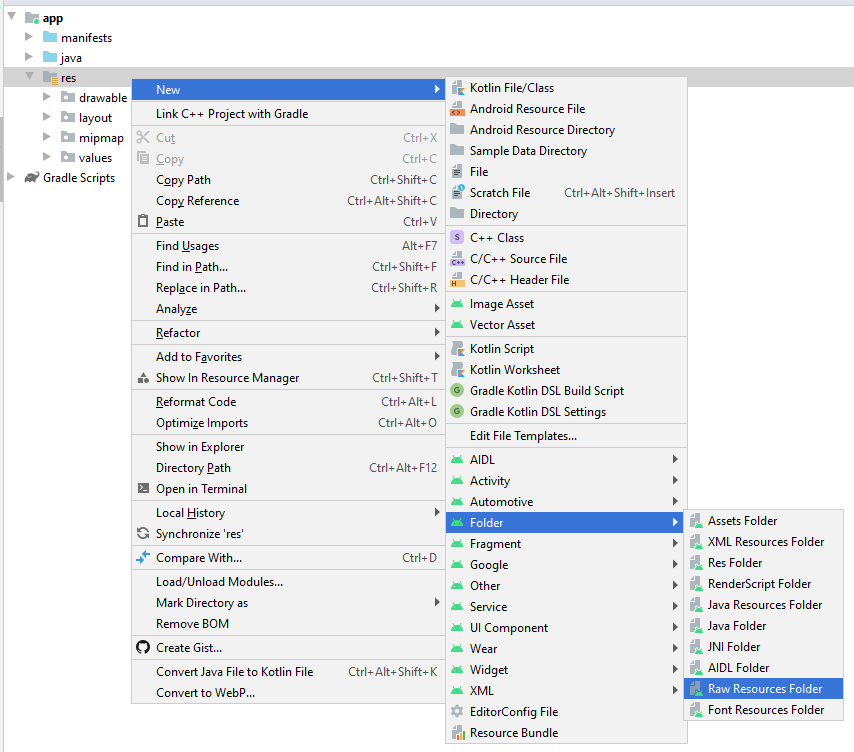
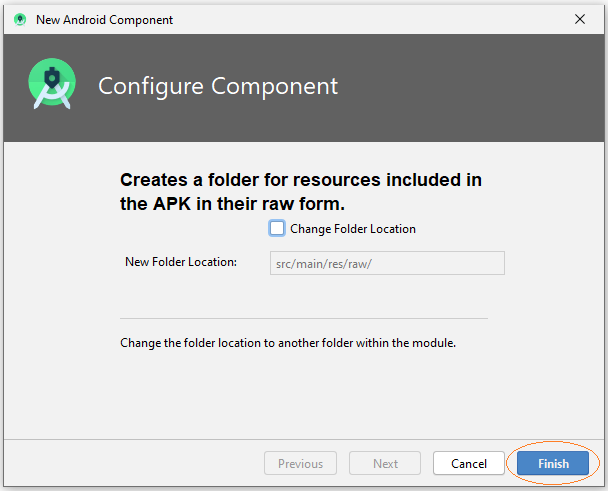
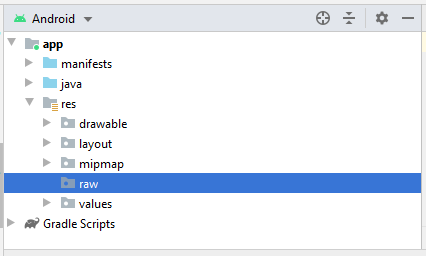
Tôi tạo thêm một vài file json trong thư mục 'raw', các file này sẽ tham gia vào một các ví dụ trong tài liệu này.
Các nguồn cung cấp dữ liệu JSON có thể từ file hoặc URL,... Ở cuối tài liệu bạn có thể xem ví dụ lấy dữ liệu JSON từ URL, phân tích và hiển thị trên một ứng dụng Android.
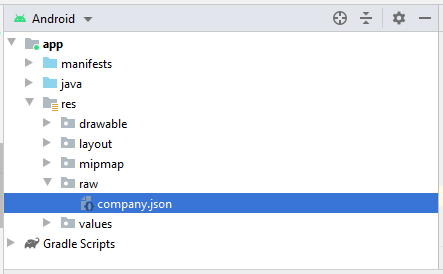
company.json
{
"id": 111 ,
"name":"Microsoft",
"websites": [
"http://microsoft.com",
"http://msn.com",
"http://hotmail.com"
],
"address":{
"street":"1 Microsoft Way",
"city":"Redmond"
}
}
Thiết kế activity_main.xml:
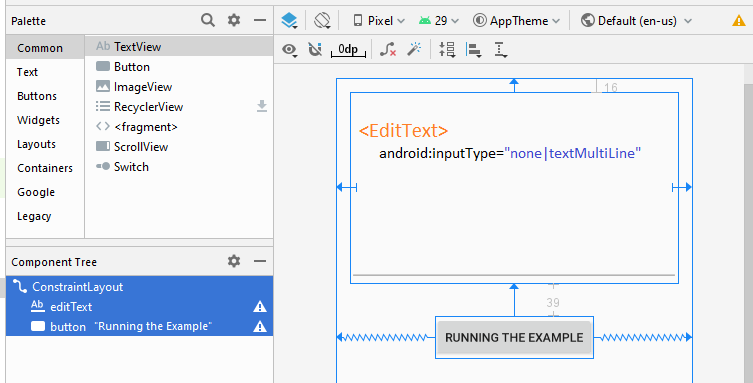
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="220dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="none|textMultiLine"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="39dp"
android:text="Running the Example"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
</androidx.constraintlayout.widget.ConstraintLayout>
4. Java Beans classes
Một số class tham gia vào các ví dụ:
Address.java
package org.o7planning.jsontutorial.beans;
public class Address {
private String street;
private String city;
public Address() {
}
public Address(String street, String city) {
this.street = street;
this.city = city;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
@Override
public String toString() {
return street + ", " + city;
}
}
Company.java
package org.o7planning.jsontutorial.beans;
public class Company {
private int id;
private String name;
private String[] websites;
private Address address;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String[] getWebsites() {
return websites;
}
public void setWebsites(String[] websites) {
this.websites = websites;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("\n id:" + this.id);
sb.append("\n name:" + this.name);
if (this.websites != null) {
sb.append("\n website: ");
for (String website : this.websites) {
sb.append(website + ", ");
}
}
if (this.address != null) {
sb.append("\n address:" + this.address.toString());
}
return sb.toString();
}
}
5. Ví dụ đọc dữ liệu JSON chuyển thành đối tượng Java
Trong ví dụ này chúng ta sẽ đọc file dữ liệu JSON và chuyển nó thành đối tượng Java.
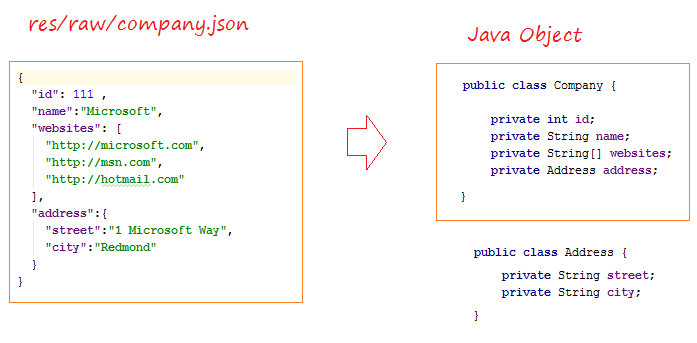
ReadJSONExample.java
package org.o7planning.jsontutorial.json;
import android.content.Context;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.o7planning.jsontutorial.R;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class ReadJSONExample {
// Read the company.json file and convert it to a java object.
public static Company readCompanyJSONFile(Context context) throws IOException,JSONException {
// Read content of company.json
String jsonText = readText(context, R.raw.company);
JSONObject jsonRoot = new JSONObject(jsonText);
int id= jsonRoot.getInt("id");
String name = jsonRoot.getString("name");
JSONArray jsonArray = jsonRoot.getJSONArray("websites");
String[] websites = new String[jsonArray.length()];
for(int i=0;i < jsonArray.length();i++) {
websites[i] = jsonArray.getString(i);
}
JSONObject jsonAddress = jsonRoot.getJSONObject("address");
String street = jsonAddress.getString("street");
String city = jsonAddress.getString("city");
Address address= new Address(street, city);
Company company = new Company();
company.setId(id);
company.setName(name);
company.setAddress(address);
company.setWebsites(websites);
return company;
}
private static String readText(Context context, int resId) throws IOException {
InputStream is = context.getResources().openRawResource(resId);
BufferedReader br= new BufferedReader(new InputStreamReader(is));
StringBuilder sb= new StringBuilder();
String s= null;
while(( s = br.readLine())!=null) {
sb.append(s);
sb.append("\n");
}
return sb.toString();
}
}
MainActivity.java
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.ReadJSONExample;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
Company company = ReadJSONExample.readCompanyJSONFile(this);
outputText.setText(company.toString());
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Chạy ví dụ:
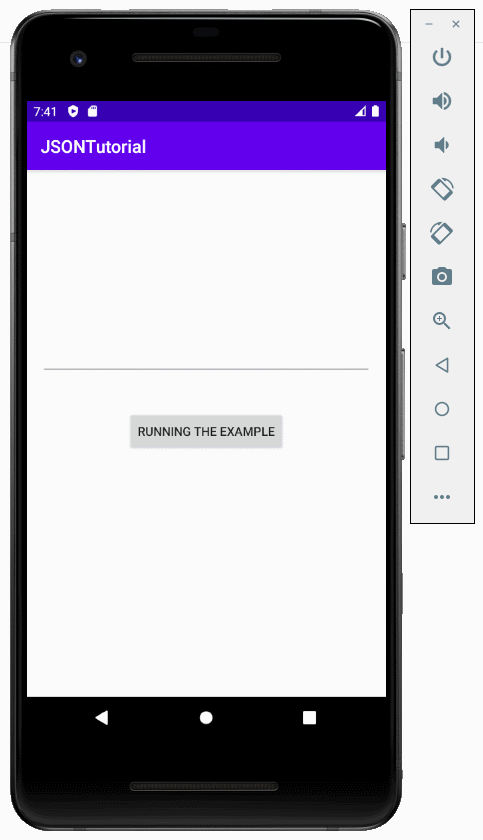
6. Ví dụ chuyển đối tượng Java thành dữ liệu JSON
Ví dụ sau chuyển một đối tượng Java thành một dữ liệu JSON.
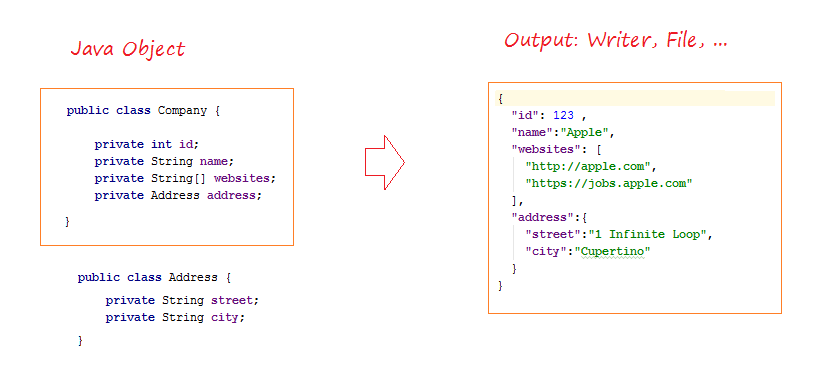
JsonWriterExample.java
package org.o7planning.jsontutorial.json;
import android.util.JsonWriter;
import org.o7planning.jsontutorial.beans.Address;
import org.o7planning.jsontutorial.beans.Company;
import java.io.IOException;
import java.io.Writer;
public class JsonWriterExample {
public static void writeJsonStream(Writer output, Company company ) throws IOException {
JsonWriter jsonWriter = new JsonWriter(output);
jsonWriter.beginObject();// begin root
jsonWriter.name("id").value(company.getId());
jsonWriter.name("name").value(company.getName());
String[] websites= company.getWebsites();
// "websites": [ ....]
jsonWriter.name("websites").beginArray(); // begin websites
for(String website: websites) {
jsonWriter.value(website);
}
jsonWriter.endArray();// end websites
// "address": { ... }
jsonWriter.name("address").beginObject(); // begin address
jsonWriter.name("street").value(company.getAddress().getStreet());
jsonWriter.name("city").value(company.getAddress().getCity());
jsonWriter.endObject();// end address
// end root
jsonWriter.endObject();
}
public static Company createCompany() {
Company company = new Company();
company.setId(123);
company.setName("Apple");
String[] websites = { "http://apple.com", "https://jobs.apple.com" };
company.setWebsites(websites);
Address address = new Address();
address.setCity("Cupertino");
address.setStreet("1 Infinite Loop");
company.setAddress(address);
return company;
}
}
MainActivity.java (2)
package org.o7planning.jsontutorial;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import org.o7planning.jsontutorial.beans.Company;
import org.o7planning.jsontutorial.json.JsonWriterExample;
import java.io.StringWriter;
public class MainActivity extends AppCompatActivity {
private EditText outputText;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.outputText = (EditText)this.findViewById(R.id.editText);
this.button = (Button) this.findViewById(R.id.button);
this.button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
runExample(view);
}
});
}
public void runExample(View view) {
try {
StringWriter output = new StringWriter();
Company company = JsonWriterExample.createCompany();
JsonWriterExample.writeJsonStream(output, company);
String jsonText = output.toString();
outputText.setText(jsonText);
} catch(Exception e) {
outputText.setText(e.getMessage());
e.printStackTrace();
}
}
}
Chạy ứng dụng:
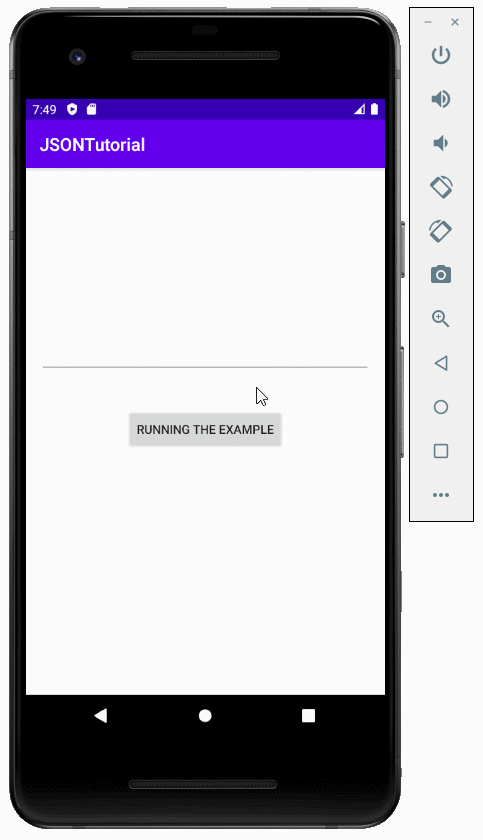
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More