Hướng dẫn và ví dụ Android Fragment
1. Android Fragment là gì?
Để thiết kế một giao diện, bạn có thể thiết kế nhiều mảnh (fragment) và ghép lại với nhau. Trong ví dụ này tôi sẽ hướng dẫn bạn làm việc với fragment.
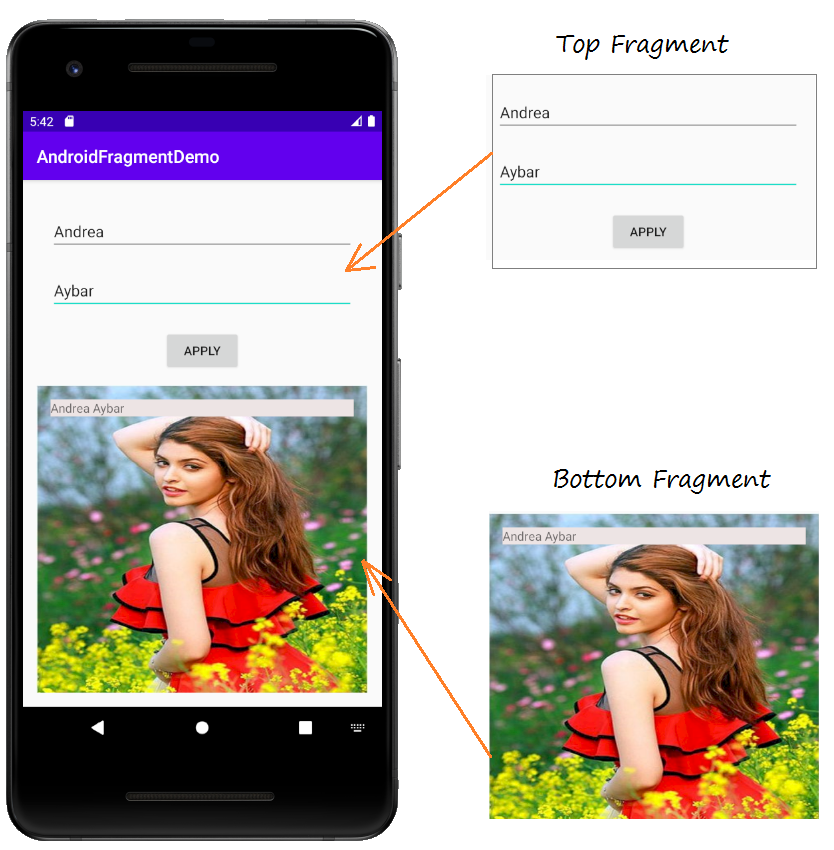
Và xử lý sự kiện tương tác giữa 2 fragment
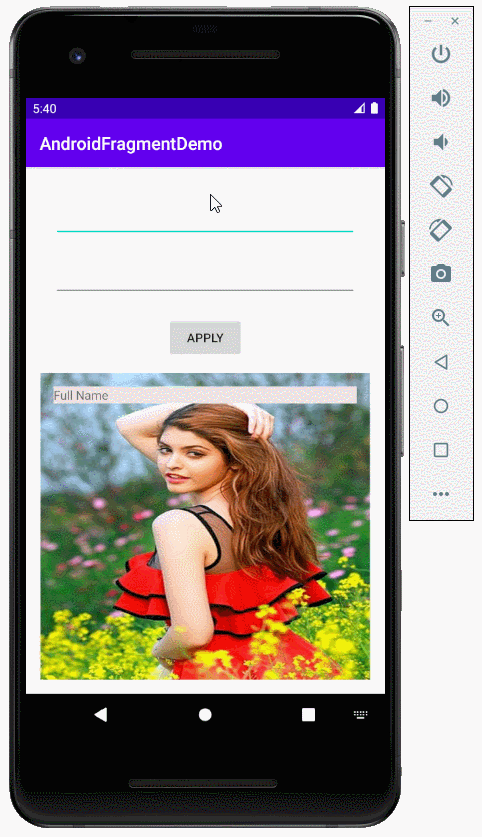
2. Ví dụ sử dụng fragment
Tạo mới một Project có tên AndroidFragmentDemo
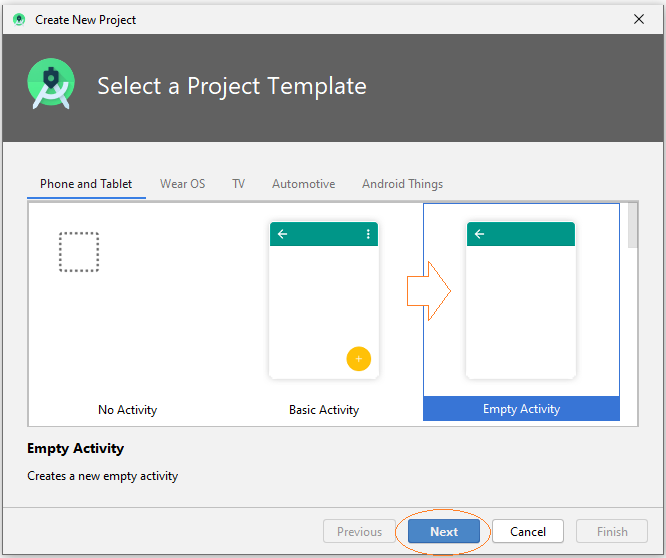
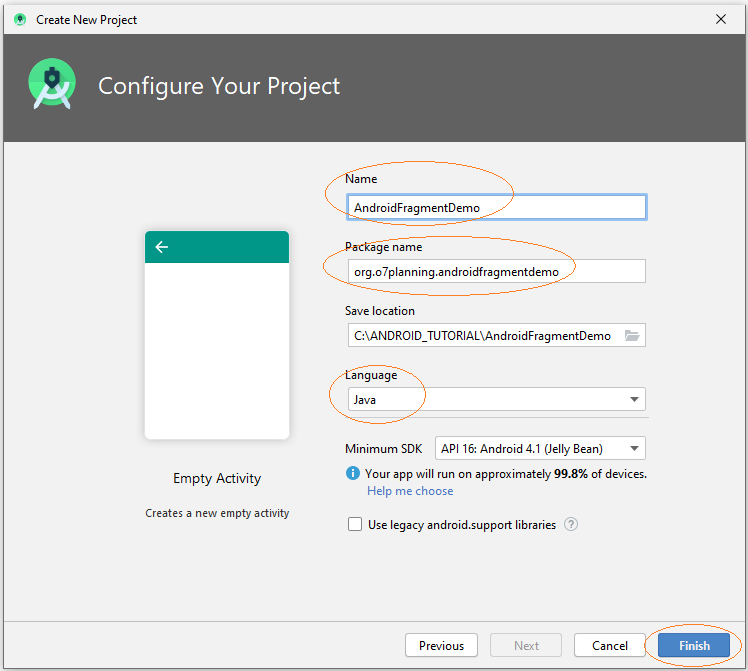
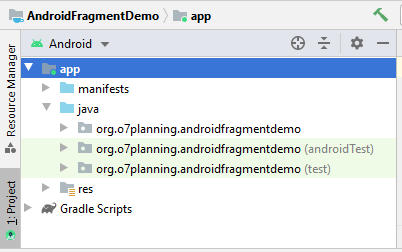
Chuẩn bị một file ảnh, chẳng hạn:
- andrea.png
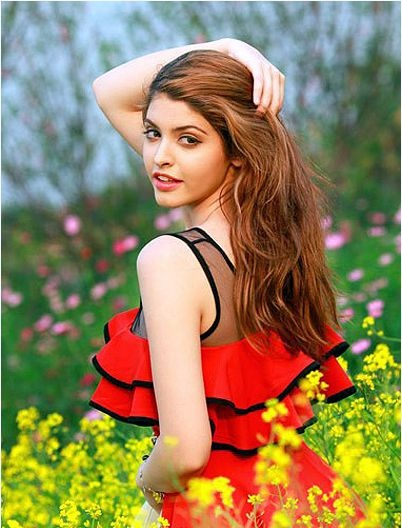
Copy và paste file andrea.jpg vào thư mục mipmap của project.
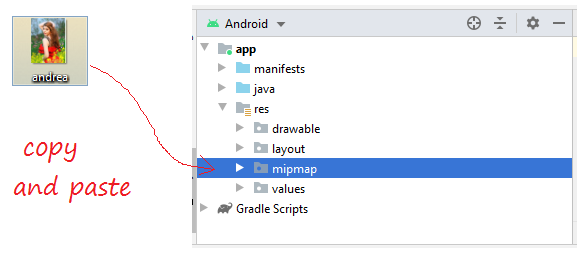
Android Studio sẽ bắt bạn chọn chất lượng ảnh sẽ được tạo ra. Chọn mipmap-mdpi đây là các ảnh với chất lượng trung bình.
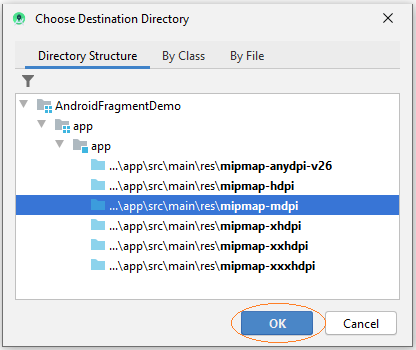
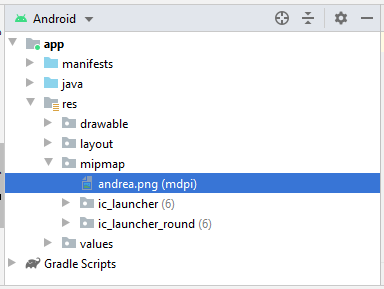
Tiếp theo chúng ta tạo file activity_top.xml:
Trên Android Studio chọn:
- File > New > Layout Resource file
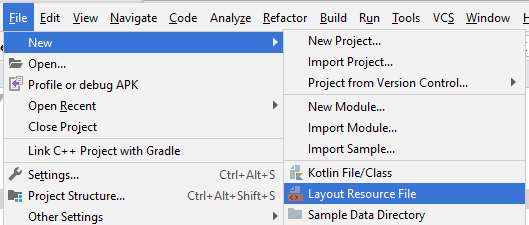
Nhập vào:
- File name: activity_top.xml
- Root element: androidx.constraintlayout.widget.ConstraintLayout
- Directory name: layout
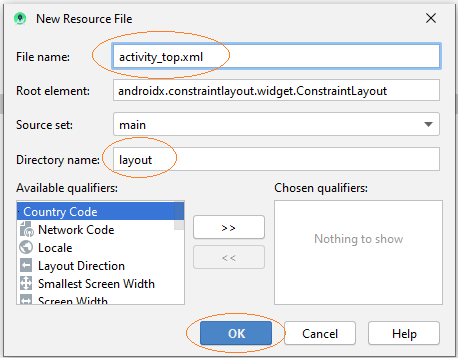
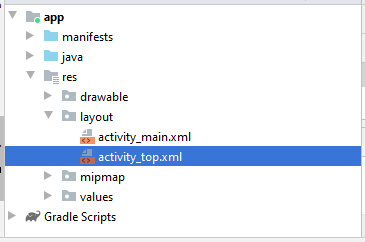
Tương tự tạo file activity_bottom.xml
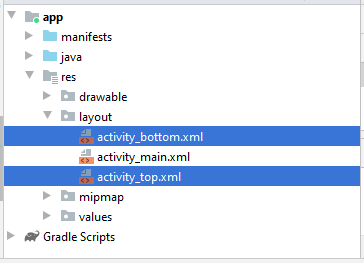
Thiết kế giao diện trên activity_top.xml
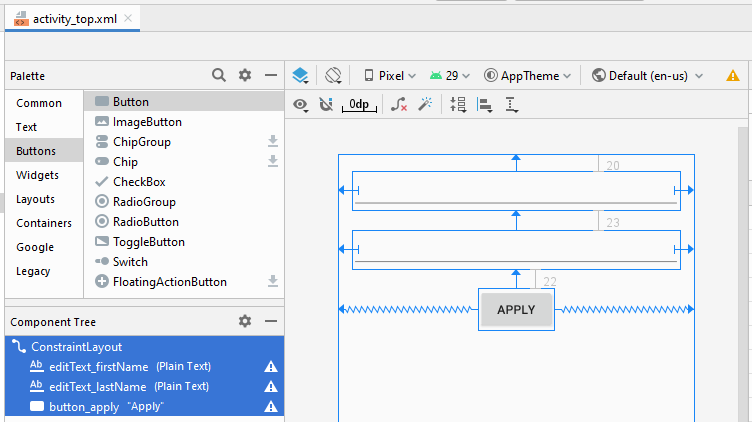
activity_top.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/editText_firstName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/editText_lastName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="23dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:ems="10"
android:inputType="textPersonName"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_firstName" />
<Button
android:id="@+id/button_apply"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="22dp"
android:text="Apply"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_lastName" />
</androidx.constraintlayout.widget.ConstraintLayout>
Thiết kế giao diện trên activity_bottom.xml
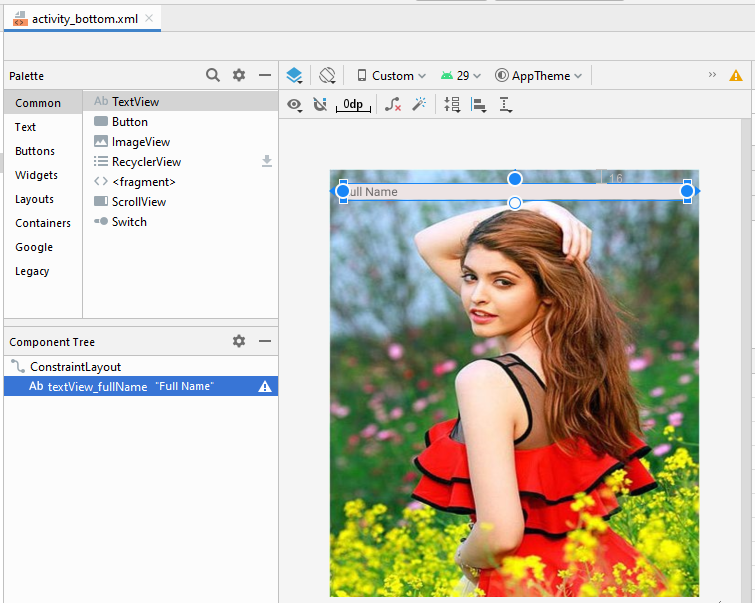
activity_bottom.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@mipmap/andrea">
<TextView
android:id="@+id/textView_fullName"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:background="#EDE4E4"
android:text="Full Name"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Nếu bạn quan tâm tới các bước để thiết kế giao diện của ứng dụng này hãy xem phần phụ lục phía cuối bài viết.
Mỗi Fragment sẽ tương ứng với một lớp trong Java. Class này mở rộng từ lớp Fragment.
Tạo 2 lớp TopFragment và BottomFragment và sửa code của nó.
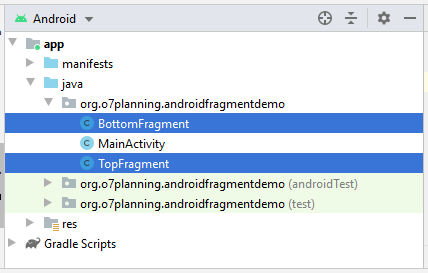
TopFragment .java
package org.o7planning.androidfragmentdemo;
import android.content.Context;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.EditText;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class TopFragment extends Fragment {
private EditText editTextFirstName;
private EditText editTextLastName;
private Button buttonApply;
private MainActivity mainActivity;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Read xml file and return View object.
// inflate(@LayoutRes int resource, @Nullable ViewGroup root, boolean attachToRoot)
View view = inflater.inflate(R.layout.activity_top, container, false);
editTextFirstName = (EditText) view.findViewById(R.id.editText_firstName);
editTextLastName = (EditText) view.findViewById(R.id.editText_lastName);
buttonApply = (Button) view.findViewById(R.id.button_apply);
buttonApply.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
applyText();
}
});
return view;
}
// Called when a fragment is first attached to its context.
@Override
public void onAttach(Context context) {
super.onAttach(context);
if (context instanceof MainActivity) {
this.mainActivity = (MainActivity) context;
}
}
private void applyText() {
String firstName = this.editTextFirstName.getText().toString();
String lastName = this.editTextLastName.getText().toString();
this.mainActivity.showText(firstName, lastName);
}
}
BottomFragment.java
package org.o7planning.androidfragmentdemo;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class BottomFragment extends Fragment {
private TextView textViewFullName;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Read xml file and return View object.
// inflate(@LayoutRes int resource, @Nullable ViewGroup root, boolean attachToRoot)
View view = inflater.inflate(R.layout.activity_bottom, container, false);
textViewFullName = (TextView) view.findViewById(R.id.textView_fullName);
return view;
}
public void showText(String firstName, String lastName) {
textViewFullName.setText(firstName + " " + lastName);
}
}
Và bây giờ, bạn cần bố trí các fragment trên giao diện chính của Activity. Mở file activity_main.xml
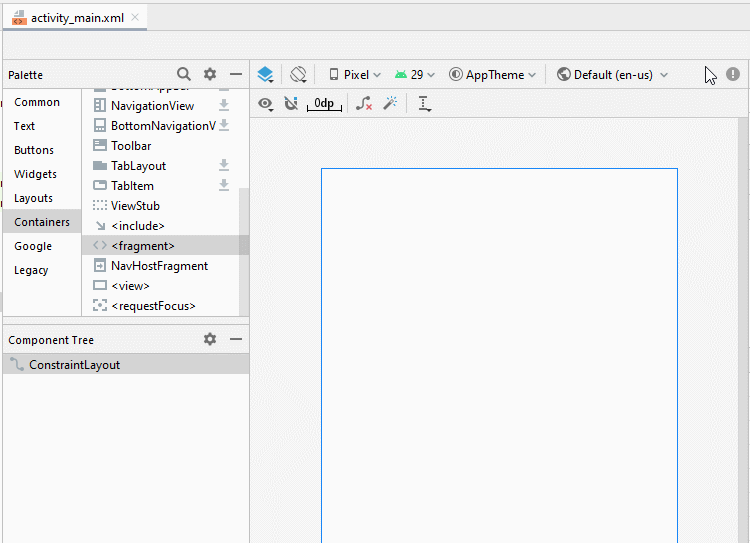
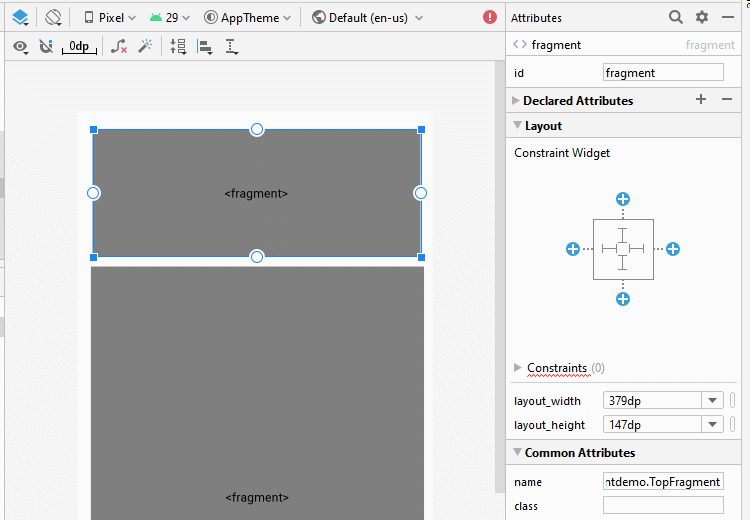
Thay đổi ID cho các fragment.
- fragment_top
- fragment_bottom
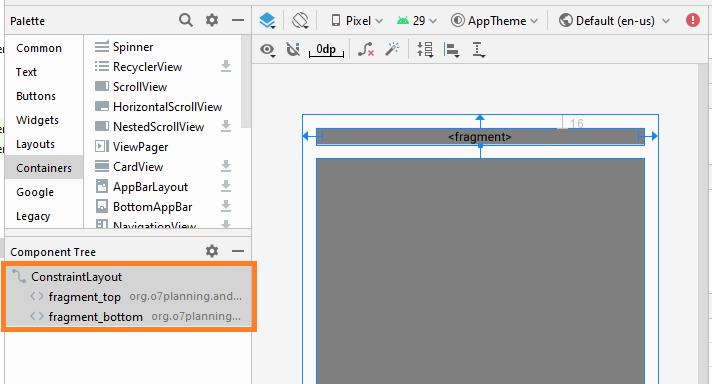
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<fragment
android:id="@+id/fragment_top"
android:name="org.o7planning.androidfragmentdemo.TopFragment"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<fragment
android:id="@+id/fragment_bottom"
android:name="org.o7planning.androidfragmentdemo.BottomFragment"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:layout_marginBottom="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/fragment_top" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.androidfragmentdemo;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void showText(String topImageText, String bottomImageText) {
BottomFragment bottomFragment
= (BottomFragment) this.getSupportFragmentManager()
.findFragmentById(R.id.fragment_bottom);
bottomFragment.showText(topImageText, bottomImageText);
}
}
Chạy ứng dụng:
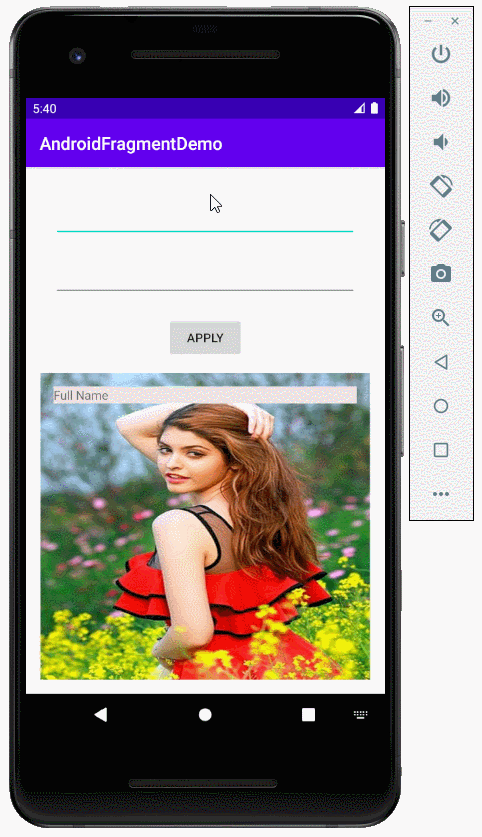
Các hướng dẫn lập trình Android
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Thiết lập SD Card cho Android Emulator
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android ToggleButton
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Ví dụ với ChipGroup và các Chip Entry
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
Show More