Hướng dẫn và ví dụ Android RatingBar
1. Android RatingBar
Trong Android, RatingBar là một điều khiển giao diện người dùng (User Interface Control) được sử dụng để nhận các đánh giá từ người dùng. RatingBar là một lớp hậu đuệ của ProgressBar nên nó có các tính năng kế thừa từ ProgressBar, nó cũng được dùng để hiển thị một đánh giá tổng hợp (trung bình cộng) từ tất cả người dùng. Về mặt giao diện RatingBar bao gồm các ngôi sao, người dùng sẽ chọn một ngôi sao để đưa ra đánh giá, ngôi sao đầu tiên tương ứng với một đánh giá thấp nhất, và ngôi sao cuối cùng tương ứng một đánh giá cao nhất.
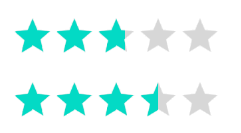
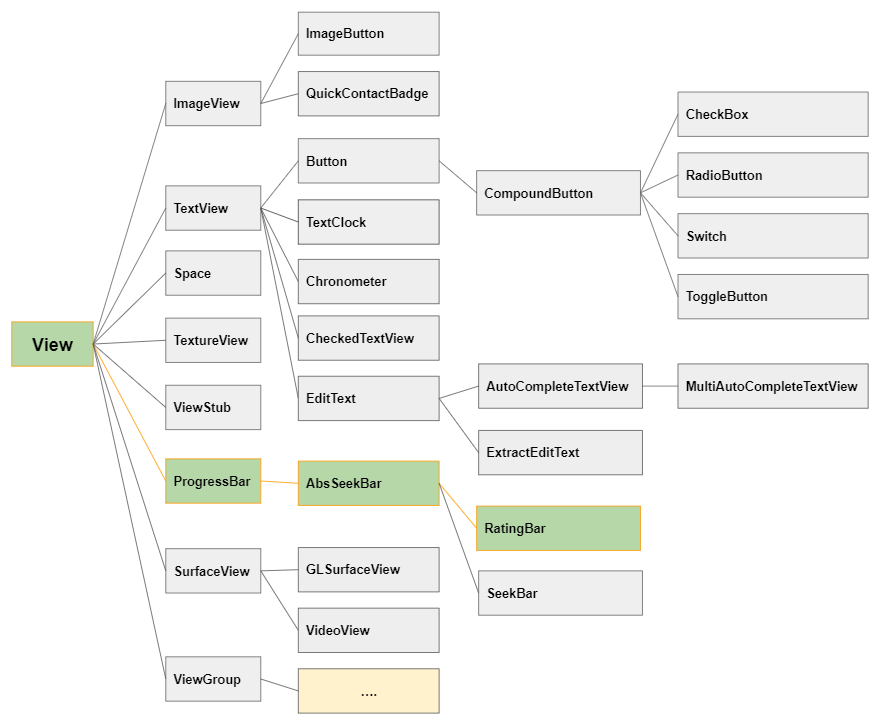
Cấu trúc của một RatingBar:
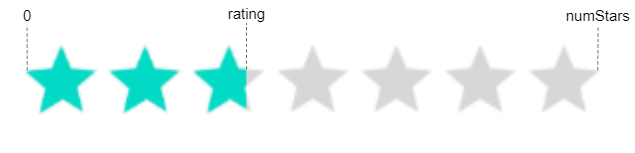
android:numStars
Thuộc tính android:numStars cho phép bạn sét đặt số lượng ngôi sao sẽ hiển thị trên RatingBar. Giá trị mặc định của nó là 5.
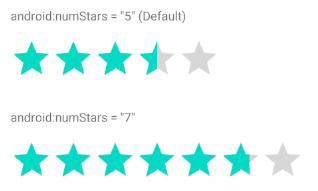
android:numStars
<!-- numStars default is 5 -->
<RatingBar
android:id="@+id/ratingBar21"
android:rating="3.5"
android:stepSize="0.1" ... />
<!-- numStars = 7 -->
<RatingBar
android:id="@+id/ratingBar22"
android:numStars="7"
android:rating="5.7"
android:stepSize="0.1" ... />
android:rating, android:stepSize
android:rating: Một giá trị biểu thị sự đánh giá của người dùng, hoặc giá trị trung bình từ tất cả các đánh giá của tất cả người dùng. Nó nằm trong khoảng (0,numStars].
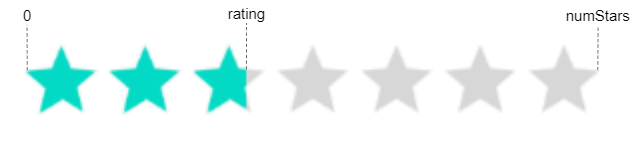
Giá trị mặc định của thuộc tính android:stepSize là 0.5, điều này của nghĩa là RatingBar sẽ chỉ hiển thị các đánh giá (Hoặc người dùng đánh giá) với giá trị 0, 0.5, 1, 1.5, 2,... Khi thay đổi giá trị của thuộc tính android:stepSite thành 0.1, RatingBar có thể hiển thị các đánh giá với giá trị: 0, 0.1, 0.2, 0.3, 0.4, 0.5, ....
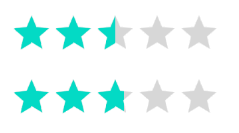
android:stepSize
<!-- android.stepSize = "0.5" (Default) -->
<RatingBar
android:id="@+id/ratingBar31"
android:rating="2.7" ... />
<!-- android.stepSize = "0.1" -->
<RatingBar
android:id="@+id/ratingBar32"
android:rating="2.7"
android:stepSize="0.1" ... />
android:isIndicator
Thuộc tính android:isIndicator = "true" được sử dụng khi bạn không muốn người dùng đưa ra đánh giá với RatingBar này. Chẳng hạn bạn muốn có một RatingBar chỉ để hiển thị một đánh giá tổng hợp (trung bình cộng) từ tất cả người dùng. Mặc định thuộc tính này có giá trị false.
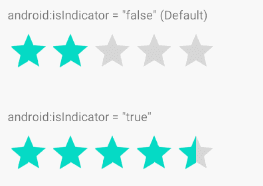
<!-- android:isIndicator = "false" (Default) -->
<RatingBar
android:id="@+id/ratingBar51"
android:rating="2" ... />
<!-- android:isIndicator = "true" (Readonly RatingBar) -->
<RatingBar
android:id="@+id/ratingBar52"
android:isIndicator="true"
android:rating="4.5" ... />
2. RatingBar Styles
Thuộc tính style là một tùy chọn của RatingBar, nó cho phép bạn sét đặt kiểu dáng cho RatingBar. Có một vài kiểu dáng sẵn có trong thư viện của Android mà bạn có thể sẵn sàng sử dụng.
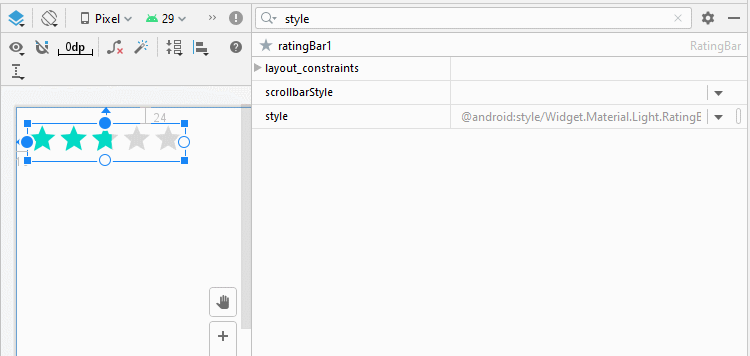
Thư viện của Android cung cấp khá nhiều kiểu dáng cho RatingBar, nhưng có khá nhiều kiểu dáng trông rất giống nhau, bạn rất khó để nhận ra sự khác biệt. Dưới đây là ví dụ với một vài kiểu dáng đẹp:
- style="@style/Widget.AppCompat.RatingBar.Indicator"
- style="@android:style/Widget.DeviceDefault.Light.RatingBar.Small"
- style="@android:style/Widget.RatingBar"
- style="@android:style/Widget.Holo.RatingBar"
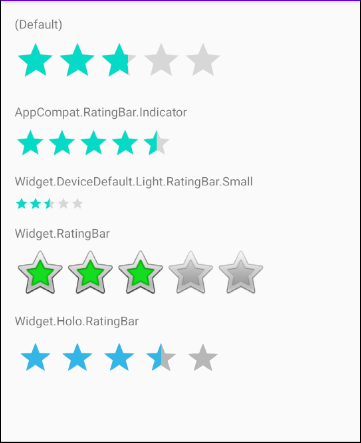
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView41"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="(Default)"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RatingBar
android:id="@+id/ratingBar41"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="2.7"
android:stepSize="0.1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView41" />
<TextView
android:id="@+id/textView42"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="AppCompat.RatingBar.Indicator"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar41" />
<RatingBar
android:id="@+id/ratingBar42"
style="@style/Widget.AppCompat.RatingBar.Indicator"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="4.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView42" />
<TextView
android:id="@+id/textView43"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.DeviceDefault.Light.RatingBar.Small"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar42" />
<RatingBar
android:id="@+id/ratingBar43"
style="@android:style/Widget.DeviceDefault.Light.RatingBar.Small"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="2.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView43" />
<TextView
android:id="@+id/textView44"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.RatingBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar43" />
<RatingBar
android:id="@+id/ratingBar44"
style="@android:style/Widget.RatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="3"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView44" />
<TextView
android:id="@+id/textView45"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Widget.Holo.RatingBar"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar44" />
<RatingBar
android:id="@+id/ratingBa45"
style="@android:style/Widget.Holo.RatingBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:rating="3.5"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView45" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. RatingBar Events
Có khá nhiều các sự kiện liên quan tới một RatingBar, nhưng các sự kiện dưới đây được sử dụng thường xuyên nhất:
- setOnClickListener(View.OnClickListener);
- setOnRatingBarChangeListener(RatingBar.OnRatingBarChangeListener);
On Click Event:
Sự kiện xẩy ra khi người dùng nhấp (click) vào RatingBar, cũng giống như hành động của người dùng khi nhấp vào một Button.
ratingBar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
RatingBar rb = (RatingBar) v;
float rating = rb.getRating();
// Your code ...
}
});
On RatingBar Changed Event
Sự kiện xẩy ra khi giá trị của rating thay đổi, do hành động của người dùng hoặc do tác dụng của việc gọi phương thức ratingBar.setRating(newRating), ..
ratingBar.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float newRating, boolean fromUser) {
// Your code
}
});
4. Ví dụ RatingBar
OK, Bây giờ chúng ta sẽ thực thực hành với một ví dụ đơn giản sử dụng RatingBar. Trong ví dụ này RatingBar thứ nhất tiếp nhận các đánh giá từ người dùng. RatingBar thứ 2 hiển thị một đánh giá tổng hợp (Trung bình cộng) từ tất cả người dùng.
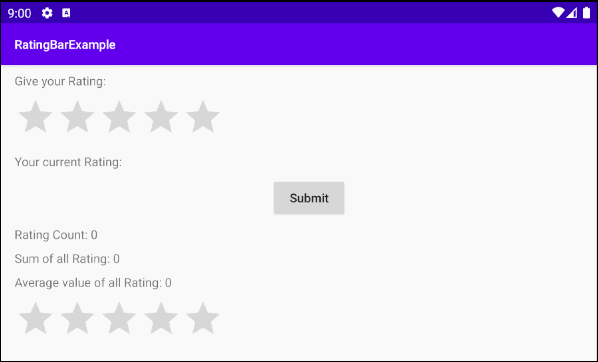
Trên Android Studio tạo mới project:
- File > New > New Project > Empty Activity
- Name: RatingBarExample
- Package name: org.o7planning.ratingbarexample
- Language: Java
Giao diện của ứng dụng:
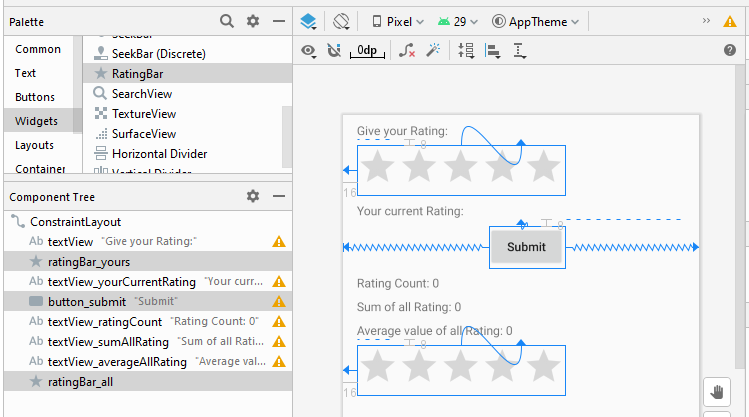
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Give your Rating:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RatingBar
android:id="@+id/ratingBar_yours"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView_yourCurrentRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Your current Rating:"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/ratingBar_yours" />
<Button
android:id="@+id/button_submit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Submit"
android:textAllCaps="false"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.52"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_yourCurrentRating" />
<TextView
android:id="@+id/textView_ratingCount"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Rating Count: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button_submit" />
<TextView
android:id="@+id/textView_sumAllRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Sum of all Rating: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_ratingCount" />
<TextView
android:id="@+id/textView_averageAllRating"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Average value of all Rating: 0"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_sumAllRating" />
<RatingBar
android:id="@+id/ratingBar_all"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="8dp"
android:isIndicator="true"
android:stepSize="0.1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView_averageAllRating" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.example.ratingbarexample;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RatingBar;
import android.widget.TextView;
import java.util.ArrayList;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private RatingBar ratingBarYours;
private RatingBar ratingBarAll;
private Button buttonSubmit;
private TextView textViewYourCurrentRating;
private TextView textViewRatingCount;
private TextView textViewSumAllRating;
private TextView textViewAverageAllRating;
private List<Float> allRatings = new ArrayList<Float>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//
this.buttonSubmit = (Button) this.findViewById(R.id.button_submit);
this.ratingBarYours = (RatingBar) this.findViewById(R.id.ratingBar_yours);
this.ratingBarAll = (RatingBar) this.findViewById(R.id.ratingBar_all);
this.textViewYourCurrentRating = (TextView) this.findViewById(R.id.textView_yourCurrentRating);
this.textViewRatingCount= (TextView) this.findViewById(R.id.textView_ratingCount);
this.textViewSumAllRating= (TextView) this.findViewById(R.id.textView_sumAllRating);
this.textViewAverageAllRating= (TextView) this.findViewById(R.id.textView_averageAllRating);
this.ratingBarYours.setOnRatingBarChangeListener(new RatingBar.OnRatingBarChangeListener() {
@Override
public void onRatingChanged(RatingBar ratingBar, float rating, boolean fromUser) {
textViewYourCurrentRating.setText("Your current Rating: " + rating);
}
});
this.buttonSubmit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
doSubmit();
}
});
}
private void doSubmit() {
float rating = this.ratingBarYours.getRating();
this.allRatings.add(rating);
int ratingCount = this.allRatings.size();
float ratingSum = 0f;
for(Float r: this.allRatings) {
ratingSum += r;
}
float averageRating = ratingSum / ratingCount;
this.textViewRatingCount.setText("Rating Count: " + ratingCount);
this.textViewSumAllRating.setText("Sum off all Rating: " + ratingSum);
this.textViewAverageAllRating.setText("Average value off all Rating: " + averageRating);
float ratingAll = this.ratingBarAll.getNumStars() * averageRating / this.ratingBarYours.getNumStars() ;
this.ratingBarAll.setRating(ratingAll);
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More