Hướng dẫn và ví dụ Android TimePickerDialog
1. Android TimePickerDialog
Android TimePickerDialog đặt một TimePicker trên một Dialog, nó cho phép người dùng lựa chọn một thời gian (Time). Về cơ bản không có sự khác biệt đáng kể về việc sử dụng giữa TimePickerDialog và TimePicker, nếu bạn muốn tiết kiệm không gian của ứng dụng hãy sử dụng TimePickerDialog.
TimePickerDialog được tạo ra trong thời gian chạy của ứng dụng, khi mà người dùng cần nó để chọn một thời gian. Bạn có thể tạo và hiển thị nó bằng mã Java:
TimePickerDialog (Clock Mode) (Default)
boolean is24HView = true;
int selectedHour = 10;
int selectedMinute = 20;
// Time Set Listener.
TimePickerDialog.OnTimeSetListener timeSetListener = new TimePickerDialog.OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker view, int hourOfDay, int minute) {
editTextTime.setText(hourOfDay + ":" + minute );
lastSelectedHour = hourOfDay;
lastSelectedMinute = minute;
}
};
// Create TimePickerDialog:
TimePickerDialog timePickerDialog = new TimePickerDialog(this,
timeSetListener, lastSelectedHour, lastSelectedMinute, is24HView);
// Show
timePickerDialog.show();
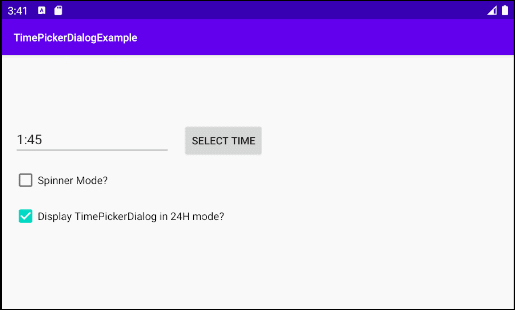
TimePickerDialog (Spinner Mode)
boolean is24HView = true;
int selectedHour = 10;
int selectedMinute = 20;
// Time Set Listener.
TimePickerDialog.OnTimeSetListener timeSetListener = new TimePickerDialog.OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker view, int hourOfDay, int minute) {
editTextTime.setText(hourOfDay + ":" + minute );
lastSelectedHour = hourOfDay;
lastSelectedMinute = minute;
}
};
// Create TimePickerDialog:
TimePickerDialog timePickerDialog = new TimePickerDialog(this,
android.R.style.Theme_Holo_Light_Dialog_NoActionBar,
timeSetListener, lastSelectedHour, lastSelectedMinute, is24HView);
// Show
timePickerDialog.show();
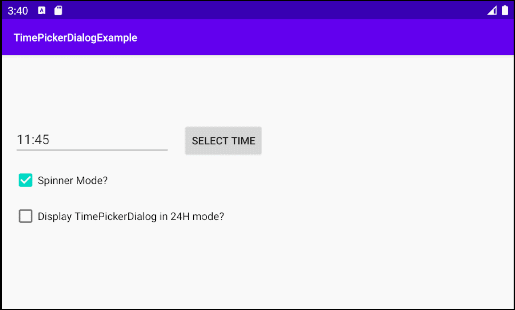
2. Ví dụ TimePickerDialog
Xem trước ví dụ:
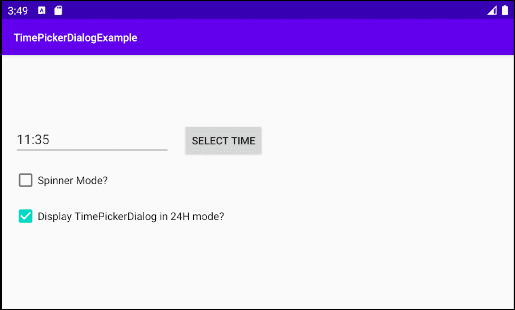
24H View Mode
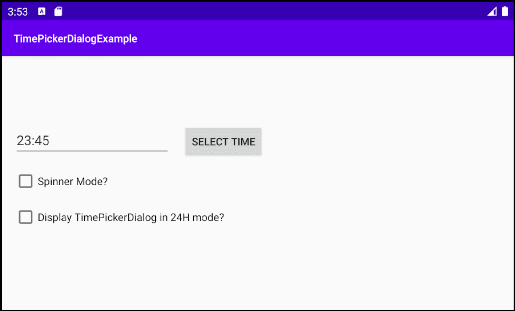
12H View Mode
Trên Android Studio tạo mới một project:
- File > New > New Project > Empty Activity
- Name: TimePickerDialogExample
- Package name: org.o7planning.timepickerdialogexample
- Language: Java
Giao diện của ứng dụng:
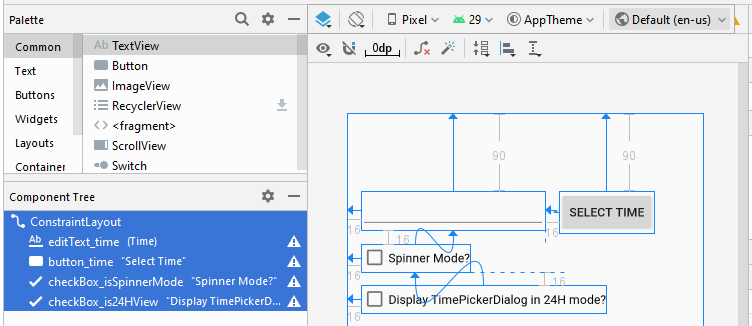
activity_main.java
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="90dp"
android:ems="10"
android:inputType="time"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="90dp"
android:text="Select Time"
app:layout_constraintStart_toEndOf="@+id/editText_time"
app:layout_constraintTop_toTopOf="parent" />
<CheckBox
android:id="@+id/checkBox_isSpinnerMode"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:text="Spinner Mode?"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText_time" />
<CheckBox
android:id="@+id/checkBox_is24HView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:text="Display TimePickerDialog in 24H mode?"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/checkBox_isSpinnerMode" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package org.o7planning.timepickerdialogexample;
import androidx.appcompat.app.AppCompatActivity;
import android.app.TimePickerDialog;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.TimePicker;
import java.util.Calendar;
public class MainActivity extends AppCompatActivity {
private EditText editTextTime;
private Button buttonTime;
private CheckBox checkBoxIsSpinnerMode;
private CheckBox checkBoxIs24HView;
private int lastSelectedHour = -1;
private int lastSelectedMinute = -1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.editTextTime = (EditText) this.findViewById(R.id.editText_time);
this.buttonTime = (Button) this.findViewById(R.id.button_time);
this.checkBoxIsSpinnerMode = (CheckBox) this.findViewById(R.id.checkBox_isSpinnerMode);
this.checkBoxIs24HView = (CheckBox) this.findViewById(R.id.checkBox_is24HView);
this.buttonTime.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
buttonSelectTime();
}
});
}
private void buttonSelectTime() {
if(this.lastSelectedHour == -1) {
// Get Current Time
final Calendar c = Calendar.getInstance();
this.lastSelectedHour = c.get(Calendar.HOUR_OF_DAY);
this.lastSelectedMinute = c.get(Calendar.MINUTE);
}
final boolean is24HView = this.checkBoxIs24HView.isChecked();
final boolean isSpinnerMode = this.checkBoxIsSpinnerMode.isChecked();
// Time Set Listener.
TimePickerDialog.OnTimeSetListener timeSetListener = new TimePickerDialog.OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker view, int hourOfDay, int minute) {
editTextTime.setText(hourOfDay + ":" + minute );
lastSelectedHour = hourOfDay;
lastSelectedMinute = minute;
}
};
// Create TimePickerDialog:
TimePickerDialog timePickerDialog = null;
// TimePicker in Spinner Mode:
if(isSpinnerMode) {
timePickerDialog = new TimePickerDialog(this,
android.R.style.Theme_Holo_Light_Dialog_NoActionBar,
timeSetListener, lastSelectedHour, lastSelectedMinute, is24HView);
}
// TimePicker in Clock Mode (Default):
else {
timePickerDialog = new TimePickerDialog(this,
timeSetListener, lastSelectedHour, lastSelectedMinute, is24HView);
}
// Show
timePickerDialog.show();
}
}
Các hướng dẫn lập trình Android
- Cấu hình Android Emulator trong Android Studio
- Hướng dẫn và ví dụ Android ToggleButton
- Tạo một File Finder Dialog đơn giản trong Android
- Hướng dẫn và ví dụ Android TimePickerDialog
- Hướng dẫn và ví dụ Android DatePickerDialog
- Bắt đầu với Android cần những gì?
- Cài đặt Android Studio trên Windows
- Cài đặt Intel® HAXM cho Android Studio
- Hướng dẫn và ví dụ Android AsyncTask
- Hướng dẫn và ví dụ Android AsyncTaskLoader
- Hướng dẫn lập trình Android cho người mới bắt đầu - Các ví dụ cơ bản
- Làm sao biết số số điện thoại của Android Emulator và thay đổi nó
- Hướng dẫn và ví dụ Android TextInputLayout
- Hướng dẫn và ví dụ Android CardView
- Hướng dẫn và ví dụ Android ViewPager2
- Lấy số điện thoại trong Android sử dụng TelephonyManager
- Hướng dẫn và ví dụ Android Phone Call
- Hướng dẫn và ví dụ Android Wifi Scanning
- Hướng dẫn lập trình Android Game 2D cho người mới bắt đầu
- Hướng dẫn và ví dụ Android DialogFragment
- Hướng dẫn và ví dụ Android CharacterPickerDialog
- Hướng dẫn lập trình Android cho người mới bắt đầu - Hello Android
- Hướng dẫn sử dụng Android Device File Explorer
- Bật tính năng USB Debugging trên thiết bị Android
- Hướng dẫn và ví dụ Android UI Layouts
- Hướng dẫn và ví dụ Android SMS
- Hướng dẫn lập trình Android với Database SQLite
- Hướng dẫn và ví dụ Google Maps Android API
- Hướng dẫn chuyển văn bản thành lời nói trong Android
- Hướng dẫn và ví dụ Android Space
- Hướng dẫn và ví dụ Android Toast
- Tạo một Android Toast tùy biến
- Hướng dẫn và ví dụ Android SnackBar
- Hướng dẫn và ví dụ Android TextView
- Hướng dẫn và ví dụ Android TextClock
- Hướng dẫn và ví dụ Android EditText
- Hướng dẫn và ví dụ Android TextWatcher
- Định dạng số thẻ tín dụng với Android TextWatcher
- Hướng dẫn và ví dụ Android Clipboard
- Tạo một File Chooser đơn giản trong Android
- Hướng dẫn và ví dụ Android AutoCompleteTextView và MultiAutoCompleteTextView
- Hướng dẫn và ví dụ Android ImageView
- Hướng dẫn và ví dụ Android ImageSwitcher
- Hướng dẫn và ví dụ Android ScrollView và HorizontalScrollView
- Hướng dẫn và ví dụ Android WebView
- Hướng dẫn và ví dụ Android SeekBar
- Hướng dẫn và ví dụ Android Dialog
- Hướng dẫn và ví dụ Android AlertDialog
- Hướng dẫn và ví dụ Android RatingBar
- Hướng dẫn và ví dụ Android ProgressBar
- Hướng dẫn và ví dụ Android Spinner
- Hướng dẫn và ví dụ Android Button
- Hướng dẫn và ví dụ Android Switch
- Hướng dẫn và ví dụ Android ImageButton
- Hướng dẫn và ví dụ Android FloatingActionButton
- Hướng dẫn và ví dụ Android CheckBox
- Hướng dẫn và ví dụ Android RadioGroup và RadioButton
- Hướng dẫn và ví dụ Android Chip và ChipGroup
- Sử dụng các tài sản ảnh và biểu tượng của Android Studio
- Thiết lập SD Card cho Android Emulator
- Ví dụ với ChipGroup và các Chip Entry
- Làm sao thêm thư viện bên ngoài vào dự án Android trong Android Studio?
- Làm sao loại bỏ các quyền đã cho phép trên ứng dụng Android
- Làm sao loại bỏ các ứng dụng ra khỏi Android Emulator?
- Hướng dẫn và ví dụ Android LinearLayout
- Hướng dẫn và ví dụ Android TableLayout
- Hướng dẫn và ví dụ Android FrameLayout
- Hướng dẫn và ví dụ Android QuickContactBadge
- Hướng dẫn và ví dụ Android StackView
- Hướng dẫn và ví dụ Android Camera
- Hướng dẫn và ví dụ Android MediaPlayer
- Hướng dẫn và ví dụ Android VideoView
- Phát hiệu ứng âm thanh trong Android với SoundPool
- Hướng dẫn lập trình mạng trong Android - Android Networking
- Hướng dẫn xử lý JSON trong Android
- Lưu trữ dữ liệu trên thiết bị với Android SharedPreferences
- Hướng dẫn lập trình Android với bộ lưu trữ trong (Internal Storage)
- Hướng dẫn lập trình Android với bộ lưu trữ ngoài (External Storage)
- Hướng dẫn sử dụng Intent trong Android
- Ví dụ về một Android Intent tường minh, gọi một Intent khác
- Ví dụ về Android Intent không tường minh, mở một URL, gửi một email
- Hướng dẫn sử dụng Service trong Android
- Hướng dẫn sử dụng thông báo trong Android - Android Notification
- Hướng dẫn và ví dụ Android DatePicker
- Hướng dẫn và ví dụ Android TimePicker
- Hướng dẫn và ví dụ Android Chronometer
- Hướng dẫn và ví dụ Android OptionMenu
- Hướng dẫn và ví dụ Android ContextMenu
- Hướng dẫn và ví dụ Android PopupMenu
- Hướng dẫn và ví dụ Android Fragment
- Hướng dẫn và ví dụ Android ListView
- Android ListView với Checkbox sử dụng ArrayAdapter
- Hướng dẫn và ví dụ Android GridView
Show More